用c语言结构题输入若干个学生的学号、姓名和成绩,用子函数实现对学生的成绩进行排序,并按成绩由高到低的次序输出学生的学号、姓名和成绩。
时间: 2023-09-06 22:08:51 浏览: 114
好的,让我来为您解答这个问题。
首先,我们需要定义一个结构体来表示一个学生的信息,包括学号、姓名和成绩。代码如下:
```c
struct student {
int id; // 学号
char name[20]; // 姓名
int score; // 成绩
};
```
接下来,我们需要输入若干个学生的信息,并将它们存储在一个数组中。代码如下:
```c
#define N 100 // 最大学生数
int n; // 学生数
struct student stu[N];
// 输入学生信息
void input()
{
printf("请输入学生数(不超过 %d 个):", N);
scanf("%d", &n);
for (int i = 0; i < n; i++) {
printf("请输入第 %d 个学生的学号、姓名和成绩:", i + 1);
scanf("%d %s %d", &stu[i].id, stu[i].name, &stu[i].score);
}
}
```
接下来,我们需要定义一个函数,用来比较两个学生的成绩大小。这个函数将用于排序操作。代码如下:
```c
// 比较函数,按成绩从高到低排序
int cmp(const void *a, const void *b)
{
return (*(struct student *)b).score - (*(struct student *)a).score;
}
```
最后,我们可以调用 qsort 函数对学生信息进行排序,并按照要求输出。代码如下:
```c
// 输出学生信息
void output()
{
printf("按成绩从高到低排序后的学生信息如下:\n");
printf("学号\t姓名\t成绩\n");
qsort(stu, n, sizeof(struct student), cmp);
for (int i = 0; i < n; i++) {
printf("%d\t%s\t%d\n", stu[i].id, stu[i].name, stu[i].score);
}
}
// 主函数
int main()
{
input();
output();
return 0;
}
```
以上就是用 C 语言结构体实现对学生信息进行排序的代码。
阅读全文
相关推荐
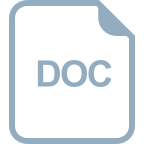
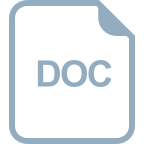
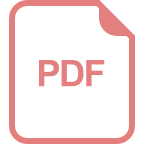
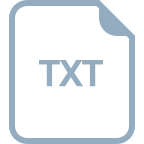
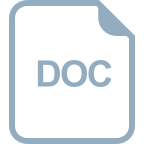
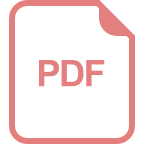
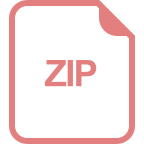
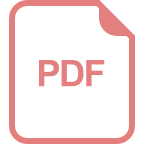
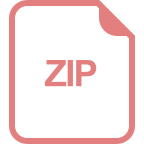
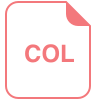
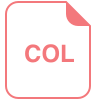
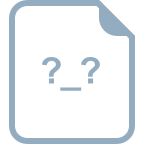
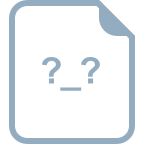