用C语言链表,输入学生姓名,学号,成绩,按姓名查找学生信息
时间: 2023-08-04 19:11:45 浏览: 433
好的,以下是一个示例程序:
```c
#include <stdio.h>
#include <stdlib.h>
#include <string.h>
// 定义学生结构体
typedef struct student {
char name[20];
int id;
float score;
struct student* next;
} Student;
// 插入学生信息
void insert(Student** head, char* name, int id, float score) {
// 创建新的学生节点
Student* new_student = (Student*)malloc(sizeof(Student));
strcpy(new_student->name, name);
new_student->id = id;
new_student->score = score;
new_student->next = NULL;
// 如果链表为空,直接将新节点作为头节点
if (*head == NULL) {
*head = new_student;
return;
}
// 找到链表尾部,将新节点插入尾部
Student* cur = *head;
while (cur->next != NULL) {
cur = cur->next;
}
cur->next = new_student;
}
// 按姓名查找学生信息
Student* find_by_name(Student* head, char* name) {
Student* cur = head;
while (cur != NULL) {
if (strcmp(cur->name, name) == 0) {
return cur;
}
cur = cur->next;
}
// 没有找到对应姓名的学生
return NULL;
}
int main() {
// 初始化链表
Student* head = NULL;
// 插入学生信息
insert(&head, "Tom", 1001, 90.5);
insert(&head, "Jerry", 1002, 85.0);
insert(&head, "Alice", 1003, 95.0);
insert(&head, "Bob", 1004, 88.5);
// 按姓名查找学生信息并输出
Student* found_student = find_by_name(head, "Alice");
if (found_student != NULL) {
printf("Name: %s\n", found_student->name);
printf("ID: %d\n", found_student->id);
printf("Score: %.1f\n", found_student->score);
} else {
printf("Student not found.\n");
}
return 0;
}
```
以上程序定义了一个 `Student` 结构体,包含学生的姓名、学号、成绩和下一个节点的指针。使用 `insert` 函数将学生信息插入链表中,使用 `find_by_name` 函数按姓名查找学生信息。在 `main` 函数中初始化链表并插入学生信息,然后查找名为 "Alice" 的学生信息并输出。
相关推荐
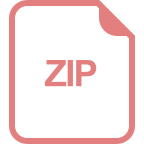
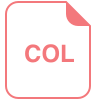
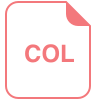
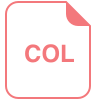
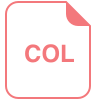
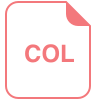









