帮我用代码写一个程序,内容是通过C语言用链表储存学生信息,信息包括学生的姓名,学号,成绩,然后还可以实现学生信息的按姓名查找,删除,插入,统计
时间: 2024-04-29 15:26:42 浏览: 55
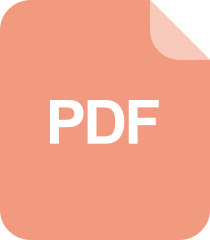
C语言使用链表实现学生信息管理系统

好的,我可以为您提供相关代码示例。
以下是用 C 语言实现链表储存学生信息的示例程序:
```c
#include <stdio.h>
#include <stdlib.h>
#include <string.h>
#define MAX_NAME_LEN 50
typedef struct Student {
char name[MAX_NAME_LEN];
int id;
int score;
struct Student* next;
} Student;
Student* create_student(char* name, int id, int score) {
Student* student = (Student*)malloc(sizeof(Student));
strcpy(student->name, name);
student->id = id;
student->score = score;
student->next = NULL;
return student;
}
Student* add_student(Student* head, char* name, int id, int score) {
Student* student = create_student(name, id, score);
if (head == NULL) {
head = student;
} else {
Student* p = head;
while (p->next != NULL) {
p = p->next;
}
p->next = student;
}
return head;
}
Student* find_student(Student* head, char* name) {
Student* p = head;
while (p != NULL) {
if (strcmp(p->name, name) == 0) {
return p;
}
p = p->next;
}
return NULL;
}
Student* remove_student(Student* head, char* name) {
if (head == NULL) {
return head;
}
Student* p = head;
if (strcmp(head->name, name) == 0) {
head = head->next;
free(p);
return head;
}
while (p->next != NULL) {
if (strcmp(p->next->name, name) == 0) {
Student* temp = p->next;
p->next = p->next->next;
free(temp);
return head;
}
p = p->next;
}
return head;
}
void print_student(Student* student) {
printf("Name: %s\nID: %d\nScore: %d\n", student->name, student->id, student->score);
}
void print_all_students(Student* head) {
Student* p = head;
while (p != NULL) {
print_student(p);
printf("\n");
p = p->next;
}
}
int count_students(Student* head) {
int count = 0;
Student* p = head;
while (p != NULL) {
count++;
p = p->next;
}
return count;
}
void destroy_all_students(Student* head) {
Student* p = head;
while (p != NULL) {
Student* temp = p;
p = p->next;
free(temp);
}
}
int main() {
Student* head = NULL;
int option;
do {
printf("Options:\n");
printf("1. Add student\n");
printf("2. Find student\n");
printf("3. Remove student\n");
printf("4. Print all students\n");
printf("5. Count students\n");
printf("6. Quit\n");
printf("Enter option number: ");
scanf("%d", &option);
switch (option) {
case 1:
printf("Enter name: ");
char name[MAX_NAME_LEN];
scanf("%s", name);
printf("Enter ID: ");
int id;
scanf("%d", &id);
printf("Enter score: ");
int score;
scanf("%d", &score);
head = add_student(head, name, id, score);
break;
case 2:
printf("Enter name: ");
char find_name[MAX_NAME_LEN];
scanf("%s", find_name);
Student* find_result = find_student(head, find_name);
if (find_result == NULL) {
printf("Not found.\n");
} else {
print_student(find_result);
}
break;
case 3:
printf("Enter name: ");
char remove_name[MAX_NAME_LEN];
scanf("%s", remove_name);
head = remove_student(head, remove_name);
break;
case 4:
print_all_students(head);
break;
case 5:
printf("Count: %d\n", count_students(head));
break;
case 6:
destroy_all_students(head);
break;
default:
printf("Invalid option.\n");
break;
}
} while (option != 6);
return 0;
}
```
该程序可以实现添加、查找、删除、打印、统计学生信息的功能。您可以根据需要进行修改和扩展。需要注意的是,该程序中没有对输入的合法性进行检查,如需用于生产环境,应添加相应的检查代码。
阅读全文
相关推荐
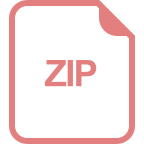






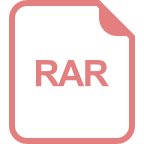
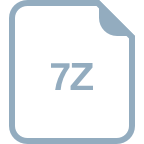
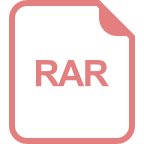
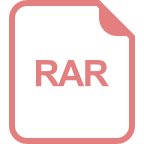
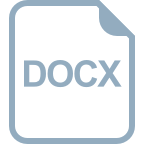
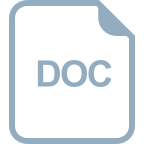
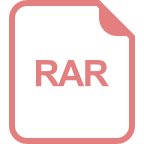
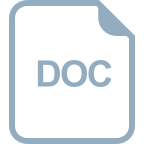
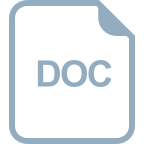
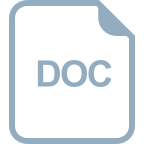