用C++代码定义一个包含学生信息(学号,姓名,成绩)的链表,使其具有如下功能
时间: 2024-05-15 17:14:57 浏览: 106
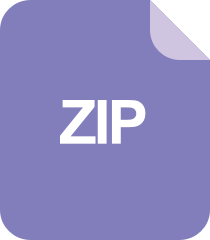
1_lower7y7_定义一个包含学生信息(学号_成绩)的顺序表和链表(二选一)_姓名_使其具有如下功能_

1. 插入学生信息
2. 删除学生信息
3. 修改学生信息
4. 查找学生信息
5. 输出所有学生信息
6. 退出程序
以下是基于C语言的实现代码:
```c
#include <stdio.h>
#include <stdlib.h>
#include <string.h>
// 学生结构体
typedef struct student {
int id; // 学号
char name[20]; // 姓名
float score; // 成绩
struct student *next; // 下一个节点指针
} student_t;
// 创建新的学生节点
student_t *new_student(int id, char *name, float score) {
student_t *node = (student_t *)malloc(sizeof(student_t));
if (node == NULL) {
printf("Error: out of memory\n");
exit(1);
}
node->id = id;
strcpy(node->name, name);
node->score = score;
node->next = NULL;
return node;
}
// 插入学生信息
void insert_student(student_t **head, student_t *node) {
if (*head == NULL) {
// 链表为空,直接将节点插入
*head = node;
} else {
// 遍历链表找到最后一个节点
student_t *ptr = *head;
while (ptr->next != NULL) {
ptr = ptr->next;
}
// 将新节点插入到链表尾部
ptr->next = node;
}
}
// 删除学生信息
void delete_student(student_t **head, int id) {
// 遍历链表查找要删除的节点
student_t *ptr = *head;
student_t *prev = NULL;
while (ptr != NULL && ptr->id != id) {
prev = ptr;
ptr = ptr->next;
}
if (ptr == NULL) {
printf("Error: student not found\n");
return;
}
// 将要删除的节点从链表中断开
if (prev == NULL) {
*head = ptr->next;
} else {
prev->next = ptr->next;
}
free(ptr);
}
// 修改学生信息
void modify_student(student_t *head, int id, char *name, float score) {
// 遍历链表查找要修改的节点
student_t *ptr = head;
while (ptr != NULL && ptr->id != id) {
ptr = ptr->next;
}
if (ptr == NULL) {
printf("Error: student not found\n");
return;
}
// 修改节点信息
strcpy(ptr->name, name);
ptr->score = score;
}
// 查找学生信息
void find_student(student_t *head, int id) {
// 遍历链表查找要查找的节点
student_t *ptr = head;
while (ptr != NULL && ptr->id != id) {
ptr = ptr->next;
}
if (ptr == NULL) {
printf("Error: student not found\n");
return;
}
// 输出节点信息
printf("ID: %d, Name: %s, Score: %.2f\n", ptr->id, ptr->name, ptr->score);
}
// 输出所有学生信息
void print_all_students(student_t *head) {
// 遍历链表输出所有节点信息
student_t *ptr = head;
while (ptr != NULL) {
printf("ID: %d, Name: %s, Score: %.2f\n", ptr->id, ptr->name, ptr->score);
ptr = ptr->next;
}
}
int main() {
student_t *head = NULL;
int choice, id;
char name[20];
float score;
while (1) {
printf("\n1. Insert student\n2. Delete student\n3. Modify student\n");
printf("4. Find student\n5. Print all students\n6. Exit\n");
printf("Enter your choice: ");
scanf("%d", &choice);
switch (choice) {
case 1:
printf("Enter student ID: ");
scanf("%d", &id);
printf("Enter student name: ");
scanf("%s", name);
printf("Enter student score: ");
scanf("%f", &score);
insert_student(&head, new_student(id, name, score));
break;
case 2:
printf("Enter student ID to delete: ");
scanf("%d", &id);
delete_student(&head, id);
break;
case 3:
printf("Enter student ID to modify: ");
scanf("%d", &id);
printf("Enter new name: ");
scanf("%s", name);
printf("Enter new score: ");
scanf("%f", &score);
modify_student(head, id, name, score);
break;
case 4:
printf("Enter student ID to find: ");
scanf("%d", &id);
find_student(head, id);
break;
case 5:
print_all_students(head);
break;
case 6:
printf("Exiting program\n");
exit(0);
default:
printf("Invalid choice\n");
}
}
return 0;
}
```
阅读全文
相关推荐
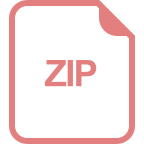
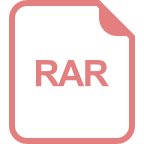














