将这段代码改为C语言代码int t, cur, first; queue<int> Q;
时间: 2023-12-20 19:03:30 浏览: 29
由于C语言中没有标准的队列容器,我们需要手动实现一个队列数据结构,或者使用第三方库来实现。以下是手动实现一个队列的示例代码:
```c
#include <stdbool.h>
#include <stdio.h>
#include <stdlib.h>
#define MAX_QUEUE_SIZE 100
int queue[MAX_QUEUE_SIZE]; // 存储队列元素
int front = 0, rear = 0; // 队首和队尾指针
void enqueue(int x) {
if ((rear + 1) % MAX_QUEUE_SIZE == front) {
// 队列已满
fprintf(stderr, "Error: queue is full\n");
exit(-1);
}
queue[rear] = x;
rear = (rear + 1) % MAX_QUEUE_SIZE;
}
int dequeue() {
if (front == rear) {
// 队列为空
fprintf(stderr, "Error: queue is empty\n");
exit(-1);
}
int x = queue[front];
front = (front + 1) % MAX_QUEUE_SIZE;
return x;
}
bool is_empty() {
return front == rear;
}
int t, cur, first;
// 假设队列中存储的是整型数据
```
在这段代码中,我们使用了一个数组queue来存储队列元素,使用front和rear指针来表示队首和队尾位置。enqueue函数用于将元素x入队,dequeue函数用于将队首元素出队并返回,is_empty函数用于判断队列是否为空。在实现BFS算法时,我们可以使用enqueue函数来将状态加入到队列中,使用dequeue函数来取出队首状态进行处理。
相关推荐
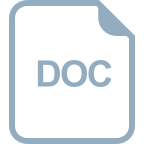
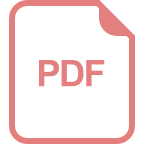











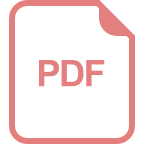
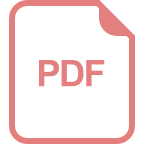
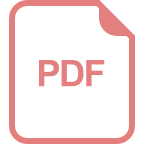
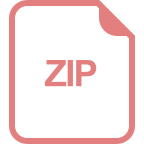