头歌Python 你是一家商店的库存管理员
时间: 2025-01-08 21:16:09 浏览: 0
### 使用 Python 实现商店库存管理系统
#### 商品类定义
为了有效地管理和操作商品信息,创建一个 `Product` 类来表示每种商品。
```python
class Product:
def __init__(self, id, name, description, price, stock):
self.id = id
self.name = name
self.description = description
self.price = price
self.stock = stock
def update_stock(self, quantity):
"""更新库存数量"""
if self.stock + quantity >= 0:
self.stock += quantity
return True
else:
print(f"{self.name} 库存不足")
return False
def display_info(self):
"""显示商品详情"""
info = f"ID: {self.id}, 名称: {self.name}, 描述: {self.description}"
info += f", 单价: ¥{self.price:.2f}, 当前库存: {self.stock}"
return info
```
#### 库存管理器类
构建一个用于处理多个产品的容器——`InventoryManager` 类,负责增删查改等功能。
```python
from typing import Dict
class InventoryManager:
def __init__(self):
self.products: Dict[int, Product] = {}
def add_product(self, product: Product):
"""添加新产品至仓库"""
if product.id not in self.products:
self.products[product.id] = product
print(f"已成功添加产品 '{product.name}' 到库存.")
else:
print(f"警告:尝试重复添加已有 ID 的产品 ({product.id})")
def remove_product_by_id(self, pid: int):
"""通过给定的产品编号移除指定条目"""
removed_item = self.products.pop(pid, None)
if removed_item is None:
print(f"未找到对应 ID({pid}) 的项目")
else:
print(f"已删除产品 '{removed_item.name}'.")
def find_product_by_name(self, pname: str) -> list:
"""按名称查找匹配项并返回列表形式的结果集"""
result = []
for p in self.products.values():
if pname.lower() in p.name.lower():
result.append(p.display_info())
return result
def adjust_inventory_levels(self, pid: int, change_amount: int):
"""调整特定项目的库存水平"""
target_prod = self.products.get(pid)
if target_prod and target_prod.update_stock(change_amount):
print(f"'{target_prod.name}' 的新库存量为 {target_prod.stock}.")
elif not target_prod:
print(f"找不到具有此 ID({pid}) 的有效产品.")
```
以上代码片段展示了如何利用面向对象编程的思想设计简单的库存管理系统框架[^1]。该系统允许管理员执行基本的操作,比如增加新的货物、查询现有物品以及修改其存储状态等。
阅读全文
相关推荐



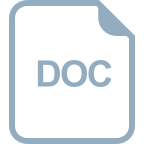














