AttributeError: 'Conv3d' object has no attribute 'input_layer'
时间: 2024-06-01 10:06:33 浏览: 8
这个错误通常是由于在调用名为 'input_layer' 的属性时,'Conv3d' 对象没有定义该属性导致的。也就是说,在使用 'Conv3d' 类的对象时,它没有 'input_layer' 属性。
要解决这个问题,你需要检查你的代码中是否有对 'Conv3d' 对象的 'input_layer' 属性的引用。如果有,则需要查看该属性是否正确定义。如果没有,则需要查看你的代码是否正确地创建了 'Conv3d' 对象。
相关问题
AttributeError: 'Conv' object has no attribute 'forward_fuse'
AttributeError: 'Conv' object has no attribute 'forward_fuse'是一个错误提示,意味着在Conv对象中没有名为forward_fuse的属性。这通常是因为代码中尝试访问了一个不存在的属性或方法。
可能的原因有:
1. Conv类确实没有定义名为forward_fuse的属性或方法。你可以检查代码中的Conv类定义,确认是否存在该属性或方法。
2. forward_fuse可能是一个拼写错误或者误用了其他类的属性或方法。你可以检查代码中对forward_fuse的使用,确认是否正确引用了该属性或方法。
3. Conv类的定义可能发生了变化,导致之前的代码无法访问forward_fuse属性。你可以检查代码中Conv类的定义,并与最新的版本进行比较,看是否有变化。
如果你能提供更多的上下文或代码片段,我可以给出更具体的帮助。
AttributeError: 'FFNNBlock' object has no attribute 'input_layer' python报错
AttributeError: 'FFNNBlock' object has no attribute 'input_layer' 是一个Python的错误提示,它表示在一个名为'FFNNBlock'的对象中找不到名为'input_layer'的属性。
这个错误通常发生在你试图访问一个对象的属性,但该属性在对象中不存在。可能有以下几个原因导致这个错误:
1. 对象没有被正确地初始化:在创建对象时,可能没有正确地初始化对象的属性。请确保在创建对象之后,正确地设置了'input_layer'属性。
2. 属性名称拼写错误:请检查属性名称是否正确拼写。Python对大小写敏感,所以属性名称必须与对象中定义的属性名称完全匹配。
3. 对象类型错误:可能你正在尝试访问一个不支持该属性的对象。请确保你正在操作正确类型的对象,并且该对象具有所需的属性。
解决这个问题的方法取决于你的具体情况。如果你是在使用第三方库或框架,可以查阅相关文档以了解正确的属性名称和使用方法。如果你自己编写了代码,可以检查你的代码逻辑并确保正确地初始化和访问属性。
相关推荐
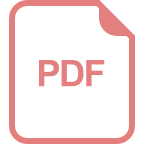
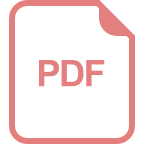
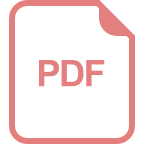












