c++中如何四舍五入保留四位小数
时间: 2024-12-10 10:51:10 浏览: 23
在C++中,你可以使用`std::round()`函数配合`std::fixed`和`std::setprecision()`来进行四舍五入并保留四位小数。首先,你需要包含`<iomanip>`头文件:
```cpp
#include <iostream>
#include <iomanip>
int main() {
double number = 3.1415926;
std::cout << std::fixed << std::setprecision(4) << round(number) << std::endl;
// 或者直接使用 C++17 的 std::to_string 和 std::round
#ifdef __cpp_lib_to_chars
std::string result = std::to_string(std::round(number));
std::cout << "Result: " << result << std::endl;
#endif
return 0;
}
```
在这里,`std::fixed`会启用固定小数点精度模式,而`std::setprecision(4)`设置小数点后的位数为4。注意,`std::round()`会将浮点数四舍五入到最接近的整数,如果你想保留小数部分并四舍五入,则需要额外处理。
相关问题
c++如何四舍五入和保留n位小数
在C++中,有几种方法可以实现四舍五入并保留指定的小数位数:
1. **std::round()函数**:这是C++标准库提供的函数,用于对浮点数进行四舍五入。例如:
```cpp
#include <cmath>
double num = 3.14159;
int rounded_num = std::round(num); // 四舍五入到最近的整数
double rounded_with_precision = static_cast<double>(rounded_num) + (num - rounded_num) / 2; // 对于保留小数位数,需要额外处理
```
2. **std::fixed和std::setprecision()**:如果你在I/O流(如cout)上设置`std::ios_base::fixed`和`std::ios_base::precision(n)`,则输出的数字会自动四舍五入到n位小数:
```cpp
std::cout << std::setprecision(2) << std::fixed << 3.14159 << std::endl; // 输出 "3.14"
```
3. **第三方库**:如果需要更复杂的功能(如银行家舍入规则),你可以考虑使用第三方库,如`boost`的`integer_rounding`。
C++四舍五入保留两位小数‘
在C++中,你可以使用`std::round()`函数或者取模运算 `%` 和整除 `/` 来实现四舍五入到两位小数。以下是两种常见方法:
1. 使用`std::round()`函数:
```cpp
#include <cmath>
double value;
// ... 获取或计算value
// 四舍五入到两位小数
double rounded_value = round(value * 100) / 100; // 如果value本身已经是浮点数
```
`std::round()`会将值四舍五入到最接近的整数,然后除以100来得到两位小数。
2. 自定义取模和整除操作:
```cpp
double value;
// ... 获取或计算value
// 将小数部分乘100并取模99(因为0.5会被向1靠近)
int decimal_part = static_cast<int>((value - floor(value)) * 100) % 100;
// 再加上整数部分,然后转换回double
rounded_value = floor(value) + (decimal_part >= 50 ? 1 : 0) + (decimal_part / 100);
```
这里假设如果小数部分大于等于50,则向前进一位。
阅读全文
相关推荐
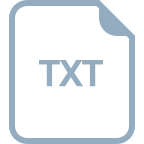
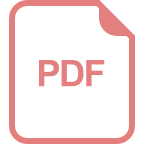













