使用遗传算法进行问题建模求解
时间: 2024-12-23 14:17:09 浏览: 3
### 使用遗传算法解决多车场车辆路径问题
#### 背景
一家物流公司位于济南,有一个配送中心负责向13个客户提供服务。每辆车的最大载重量为2吨(即2000公斤),具体客户需求及配送点间距离见下表:
| | 配送中心1 | 客户1 | 客户2 | ... | 客户13 |
|--|-------|-------|-----|--------|
| **配送中心1** | 0 | 15 | 11 | ... | 11 |
| **客户1** | 15 | 0 | 19 | ... | 20 |
| **客户2** | 11 | 19 | 0 | ... | 9 |
| ... | ... | ... | ... | ... | ... |
| **客户13** | 11 | 20 | 9 | ... | 0 |
客户物品重量分别为:564, 509, 503, 350, 297, 355, 595, 446, 292, 343, 443, 233, 227 公斤。
#### 目标
通过遗传算法设计合理的配送路线,使总配送距离最短。
#### 步骤
1. **定义染色体**
- 每条染色体表示一个可能的配送方案。
- 染色体由多个基因组成,每个基因代表一个客户的编号。
- 例如,一条染色体可以是 `[1, 3, 5, 2, 4, 6, 7, 8, 9, 10, 11, 12, 13]`,表示按此顺序访问客户。
2. **适应度函数**
- 计算每条染色体对应的总配送距离。
- 总配送距离包括从配送中心到第一个客户、各个客户之间的距离以及最后一个客户返回配送中心的距离。
- 适应度值越小,表示该方案越好。
3. **选择操作**
- 采用轮盘赌选择法或锦标赛选择法,选择适应度较高的染色体进入下一代。
4. **交叉操作**
- 选择两条父代染色体,生成子代染色体。
- 可以使用部分映射交叉(PMX)、顺序交叉(OX)等方法。
5. **变异操作**
- 对选中的染色体进行随机交换、插入或逆序等操作,增加种群多样性。
6. **终止条件**
- 达到预定的迭代次数。
- 连续若干代适应度没有显著提高。
#### 示例代码
以下是一个简单的Python示例,展示了如何实现上述步骤:
```python
import random
import numpy as np
# 定义参数
num_vehicles = 3
max_weight = 2000
distances = [
[0, 15, 11, 13, 20, 7, 8, 5, 12, 15, 7, 19, 6, 11],
[15, 0, 19, 7, 15, 19, 17, 13, 7, 14, 6, 7, 9, 20],
[11, 19, 0, 18, 20, 8, 14, 17, 6, 8, 6, 13, 18, 9],
[13, 7, 18, 0, 6, 11, 13, 14, 15, 13, 15, 11, 16, 6],
[20, 15, 20, 6, 0, 17, 14, 6, 7, 19, 15, 14, 17, 16],
[7, 19, 8, 11, 17, 0, 14, 6, 7, 19, 13, 9, 10, 12],
[8, 17, 14, 13, 14, 14, 0, 8, 8, 15, 7, 15, 12, 5],
[5, 13, 17, 14, 6, 6, 8, 0, 11, 16, 16, 13, 10, 20],
[12, 7, 6, 15, 7, 7, 8, 11, 0, 16, 10, 19, 17, 17],
[15, 14, 18, 13, 19, 19, 15, 16, 16, 0, 7, 16, 7, 19],
[7, 6, 6, 15, 15, 13, 7, 16, 10, 7, 0, 10, 17, 7],
[19, 7, 13, 11, 14, 9, 15, 13, 19, 16, 10, 0, 9, 14],
[6, 9, 18, 16, 17, 10, 12, 10, 17, 7, 17, 9, 0, 13],
[11, 20, 9, 6, 16, 12, 5, 20, 17, 19, 7, 14, 13, 0]
]
weights = [564, 509, 503, 350, 297, 355, 595, 446, 292, 343, 443, 233, 227]
def calculate_total_distance(route):
total_distance = distances[0][route[0]] # 从配送中心到第一个客户
for i in range(len(route) - 1):
total_distance += distances[route[i]][route[i + 1]]
total_distance += distances[route[-1]][0] # 最后一个客户返回配送中心
return total_distance
def generate_initial_population(pop_size):
population = []
for _ in range(pop_size):
route = list(range(1, len(weights) + 1))
random.shuffle(route)
population.append(route)
return population
def select_parents(population, fitnesses, num_parents):
parents = []
for _ in range(num_parents):
tournament = random.sample(list(zip(population, fitnesses)), 5)
best_parent = min(tournament, key=lambda x: x[1])[0]
parents.append(best_parent)
return parents
def crossover(parents, offspring_size):
offspring = []
while len(offspring) < offspring_size:
parent1, parent2 = random.sample(parents, 2)
start, end = sorted(random.sample(range(len(parent1)), 2))
child = [-1] * len(parent1)
child[start:end+1] = parent1[start:end+1]
idx = 0
for gene in parent2:
if gene not in child:
while child[idx] != -1:
idx += 1
child[idx] = gene
offspring.append(child)
return offspring
def mutate(individual, mutation_rate=0.01):
for i in range(len(individual)):
if random.random() < mutation_rate:
j = random.randint(0, len(individual) - 1)
individual[i], individual[j] = individual[j], individual[i]
return individual
def genetic_algorithm(pop_size=100, num_generations=1000, mutation_rate=0.01):
population = generate_initial_population(pop_size)
for generation in range(num_generations):
fitnesses = [calculate_total_distance(route) for route in population]
parents = select_parents(population, fitnesses, pop_size // 2)
offspring = crossover(parents, pop_size)
for i in range(len(offspring)):
offspring[i] = mutate(offspring[i], mutation_rate)
population = offspring
best_route = min(population, key=lambda x: calculate_total_distance(x))
return best_route, calculate_total_distance(best_route)
best_route, best_distance = genetic_algorithm()
print("最佳配送路线:", best_route)
print("总配送距离:", best_distance)
```
#### 结论
通过上述遗传算法,可以有效地找到一个较优的配送方案,使得总配送距离最小。
阅读全文
相关推荐
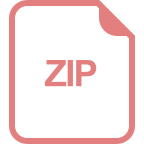
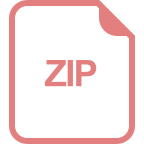
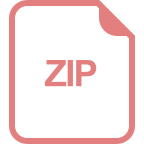
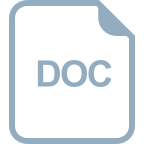
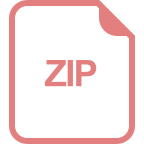
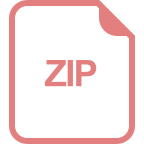
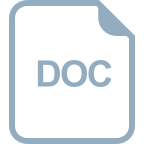
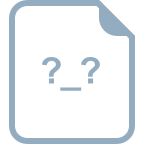
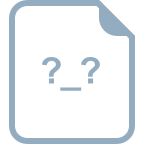
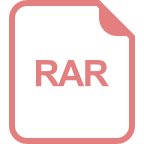
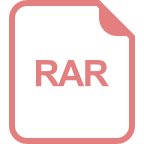
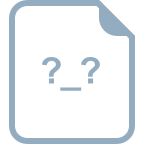
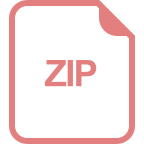
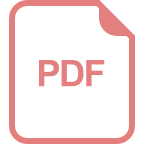
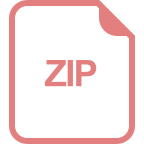