本题要求实现一个函数,可将两个相同长度列表中的元素依次相除,返回结果列表。例如[6, 12, 24] 和 [3, 4, 6] 的元素依次相除得到 2, 3, 4 ,则该函数返回 [2, 3, 4] 。该函数对异常的处理如下: 该函数应能在内部消化掉被除数为0的异常 ZeroDivisionError ,应将其结果统一为float('nan')。 对于其他的异常,统一抛出自定义的ValueError异常(包含异常信息"get_ratios called with bad arg")。 函数接口定义: def get_ratios(L1, L2) 其中 L1 和 L2 都是列表,元素类型为int。 裁判测试程序样例: # 请在这里填写答案 L1 = map(int, input().split(" ")) L2 = map(int, input().split(" ")) try: print(get_ratios(L1, L2)) except Exception as e: print(e)
时间: 2024-03-14 18:43:18 浏览: 66
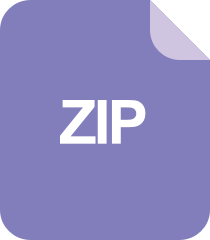
获取两个数组相同的元素或不同的元素输出
你可以使用以下代码实现该函数:
```python
def get_ratios(L1, L2):
try:
ratios = []
for i in range(len(L1)):
ratio = L1[i] / L2[i]
ratios.append(ratio)
return ratios
except ZeroDivisionError:
return [float('nan')] * len(L1)
except:
raise ValueError("get_ratios called with bad arg")
```
该函数首先尝试依次计算两个列表中对应位置上的元素的比值,并将结果存储在 `ratios` 列表中。如果在计算过程中出现了被除数为 0 的情况,就将该位置上的比值设为 `float('nan')`。如果出现其他异常,则将异常信息封装在 `ValueError` 中并抛出。最后返回 `ratios` 列表。
调用该函数的代码如下:
```python
L1 = list(map(int, input().split(" ")))
L2 = list(map(int, input().split(" ")))
try:
print(get_ratios(L1, L2))
except Exception as e:
print(e)
```
该代码首先读取两行输入,每行输入都是一串数字,使用 `map` 函数将字符串类型的数字转换为整型,并存储在 `L1` 和 `L2` 两个列表中。然后调用 `get_ratios` 函数,该函数接受两个参数,均为列表类型,用于计算两个列表对应位置上的数的比例,并将结果存储在一个新的列表中。最后使用 `try...except` 语句来捕获可能出现的异常,如果出现异常则打印异常信息。
阅读全文
相关推荐
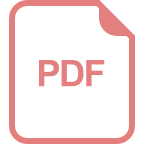

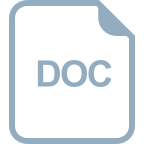
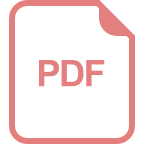
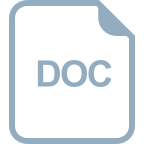
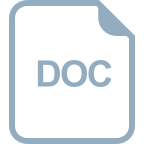
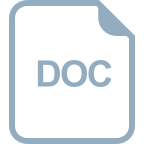
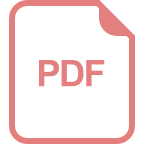
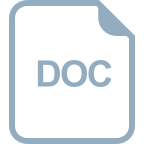
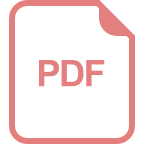
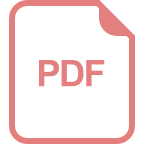
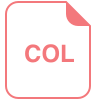
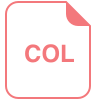
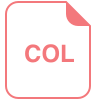
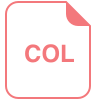
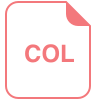
