小红拿到了一个只包含 'a' , 'b' , 'c' 三种字符的字符串。 小红想知道,这个字符串最短的、长度超过 1 的回文子串的长度是多少? 子串定义:字符串取一段连续的区间。例如"abcca"的子串有"ab"、"bcca"等,但"aca"则不是它的子串。 回文的定义:一个字符串正着读和倒着读都是相同的,那么定义它的回文的。c++
时间: 2024-04-15 18:29:55 浏览: 138
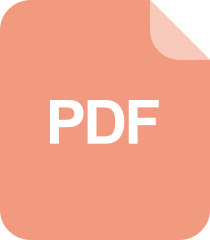
java & Android 格式化字符串详解
对于只包含 'a' , 'b' , 'c' 三种字符的字符串,我们可以通过遍历字符串的所有可能子串,判断每个子串是否为回文,并记录最小长度超过1的回文子串的长度。下面是一个C++的实现示例:
```cpp
#include <iostream>
#include <string>
using namespace std;
int shortestPalindromeLength(string s) {
int n = s.length();
int shortestLength = n + 1;
for (int i = 0; i < n; i++) {
for (int j = i + 1; j <= n; j++) {
string sub = s.substr(i, j - i);
bool isPalindrome = true;
for (int k = 0; k < sub.length() / 2; k++) {
if (sub[k] != sub[sub.length() - k - 1]) {
isPalindrome = false;
break;
}
}
if (isPalindrome && sub.length() > 1) {
shortestLength = min(shortestLength, static_cast<int>(sub.length()));
}
}
}
return shortestLength > n ? -1 : shortestLength;
}
int main() {
string s;
cout << "请输入字符串:";
cin >> s;
int shortestLength = shortestPalindromeLength(s);
if (shortestLength == -1) {
cout << "不存在长度超过1的回文子串" << endl;
} else {
cout << "最短的长度超过1的回文子串的长度为:" << shortestLength << endl;
}
return 0;
}
```
在上述代码中,我们通过两层循环遍历所有可能的子串,并使用额外的循环判断每个子串是否为回文。如果是回文且长度大于1,则更新最小长度。最后,如果最小长度仍然是初始值(n+1),则表示不存在长度超过1的回文子串,返回-1;否则,返回最小长度。
注意:这只是一种解法,可能不是最优解。在实际应用中,可以根据实际情况进行优化。
阅读全文
相关推荐
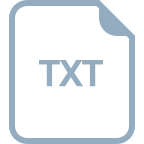
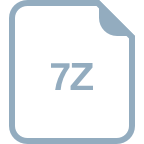












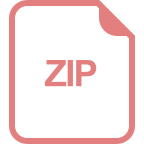
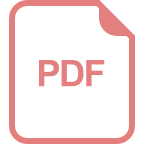
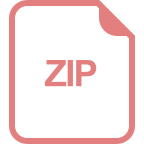