python 中sum()函数用法
时间: 2024-04-08 10:30:16 浏览: 9
`sum()`函数是Python内置函数之一,用于计算可迭代对象中元素的总和。它的基本用法如下:
```python
sum(iterable, start=0)
```
其中,`iterable`是一个可迭代对象,可以是列表、元组、集合等,`start`是可选参数,表示起始值,默认为0。
下面是一些示例:
```python
# 列表求和
numbers = [1, 2, 3, 4, 5]
total = sum(numbers)
print(total) # 输出: 15
# 元组求和
numbers = (1, 2, 3, 4, 5)
total = sum(numbers)
print(total) # 输出: 15
# 集合求和
numbers = {1, 2, 3, 4, 5}
total = sum(numbers)
print(total) # 输出: 15
# 指定起始值求和
numbers = [1, 2, 3, 4, 5]
total = sum(numbers, 10)
print(total) # 输出: 25 (10 + 1 + 2 + 3 + 4 + 5)
```
`sum()`函数在处理字符串列表时要小心,因为它会将字符串连接起来,而不是数值相加。如果要对字符串列表求和,可以使用列表推导式或`map()`函数将字符串转换为数值类型后再求和。
相关问题
python中sum函数用法
Python中的sum函数用于对一个可迭代对象中的元素进行求和操作,其语法格式为:
sum(iterable[, start])
其中,iterable表示可迭代对象,start表示可选的起始值,默认为0。例如,对于一个列表[1, 2, 3, 4, 5],可以使用sum函数求和:
>>> nums = [1, 2, 3, 4, 5]
>>> total = sum(nums)
>>> print(total)
15
需要注意的是,sum函数只能对数字类型的元素进行求和,如果可迭代对象中包含非数字类型的元素,则会抛出TypeError异常。
python中sum函数的用法
在Python中,`sum()`函数用于计算可迭代(如列表、元组、集合等)中所有元素的总和。它接受一个可迭代对象作为参数,并返回总和结果。
以下`sum()`函数的一些常见用法例:
1. 对列表进行求和:
```
numbers = [1, 2, 3, 4, 5]
total = sum(numbers)
print(total) # 输出:15
```
2. 对元组进行求和:
```python
numbers = (1, 2, 3, 4, 5)
total = sum(numbers)
print(total) # 输出:15
```
3. 对集合进行求和:
```python
numbers = {1, 2, 3, 4, 5}
total = sum(numbers)
print(total) # 输出:15
```
4. 对带有自定义对象的可迭代对象进行求和,需要提供一个可选的`key`参数来指定如何提取每个对象的值进行求和。例如,对包含学生对象的列表按照他们的分数求和:
```python
class Student:
def __init__(self, name, score):
self.name = name
self.score = score
students = [Student('Alice', 80), Student('Bob', 90), Student('Charlie', 70)]
total_score = sum(students, key=lambda x: x.score)
print(total_score) # 输出:240
```
总结一下,`sum()`函数用于计算可迭代对象中元素的总和,并且可以通过`key`参数进行自定义求和的方式。
相关推荐
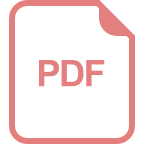
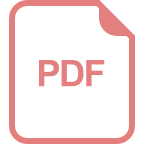












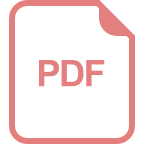