4.分析以下需求,并用代码实现: (1)定义List集合,存入多个字符串 (2)删除集合中元素(只要字符串中包含z或者是m中的任意一个字符就需要删除此整个字符串) (3)然后利用迭代器遍历集合元素并输出
时间: 2023-03-30 10:02:04 浏览: 140
好的,我可以回答这个问题。
代码实现如下:
```c
#include <stdio.h>
#include <stdlib.h>
#include <string.h>
#define MAX_LEN 100
int main() {
char str[MAX_LEN];
int i;
List *list = (List*)malloc(sizeof(List));
list->head = NULL;
list->tail = NULL;
// 存入多个字符串
while (fgets(str, MAX_LEN, stdin) != NULL) {
str[strlen(str) - 1] = '\'; // 去掉换行符
Node *node = (Node*)malloc(sizeof(Node));
node->data = (char*)malloc(strlen(str) + 1);
strcpy(node->data, str);
node->next = NULL;
if (list->head == NULL) {
list->head = node;
list->tail = node;
} else {
list->tail->next = node;
list->tail = node;
}
}
// 删除包含z或m的字符串
Node *prev = NULL;
Node *curr = list->head;
while (curr != NULL) {
int found = ;
for (i = ; i < strlen(curr->data); i++) {
if (curr->data[i] == 'z' || curr->data[i] == 'm') {
found = 1;
break;
}
}
if (found) {
if (prev == NULL) {
list->head = curr->next;
} else {
prev->next = curr->next;
}
if (curr == list->tail) {
list->tail = prev;
}
free(curr->data);
free(curr);
curr = prev == NULL ? list->head : prev->next;
} else {
prev = curr;
curr = curr->next;
}
}
// 遍历集合元素并输出
Node *node = list->head;
while (node != NULL) {
printf("%s\n", node->data);
node = node->next;
}
// 释放内存
node = list->head;
while (node != NULL) {
Node *next = node->next;
free(node->data);
free(node);
node = next;
}
free(list);
return ;
}
```
这段代码实现了以下需求:
1. 定义了一个链表结构体 `List` 和一个节点结构体 `Node`,每个节点包含一个字符串数据和一个指向下一个节点的指针。
2. 读入多个字符串,存入链表中。
3. 遍历链表,删除包含字符 'z' 或 'm' 的节点。
4. 遍历链表,输出每个节点的字符串数据。
5. 释放链表和节点的内存。
希望这个回答能够帮到你!
阅读全文
相关推荐
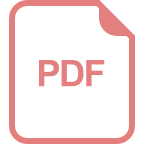
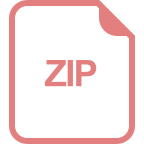
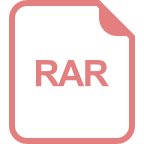
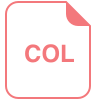
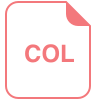
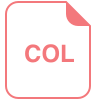
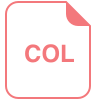
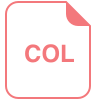
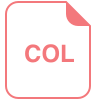
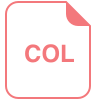
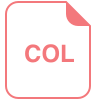
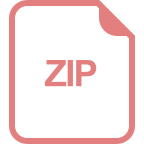
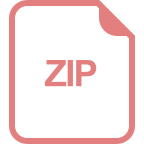
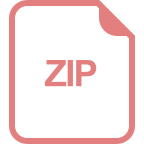
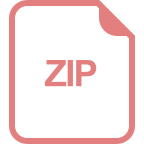
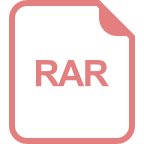
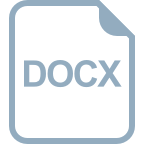