ValueError: setting an array element with a sequence.
时间: 2023-09-23 20:06:59 浏览: 121
This error occurs when you are trying to assign a sequence (such as a list or tuple) to an element in a numpy array. Numpy arrays are designed to hold elements of a specific data type and size, and cannot accommodate sequences of varying lengths or types.
To fix this error, you need to ensure that the sequence you are trying to assign to the array is of the same data type and size as the other elements in the array. You can use the numpy functions `dtype` and `shape` to check the data type and size of the array elements, and then convert the sequence to the same data type and size before assigning it to the array.
For example, if you have a numpy array with dtype `int` and shape `(3,)`, you can create a sequence of three integers and assign it to the array as follows:
```
import numpy as np
# create numpy array
arr = np.zeros(3, dtype=int)
# create sequence of three integers
seq = [1, 2, 3]
# check data type and size of array elements
print(arr.dtype) # int64
print(arr.shape) # (3,)
# convert sequence to same data type and size as array elements
seq = np.array(seq, dtype=int)
# assign sequence to array
arr[1] = seq
# check array values
print(arr) # [0 1 0]
```
In this example, the sequence `seq` is converted to a numpy array with dtype `int` and shape `(3,)` before being assigned to the second element of the `arr` array.
阅读全文
相关推荐
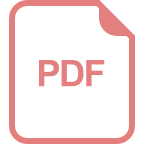
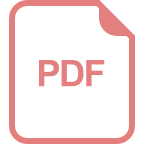
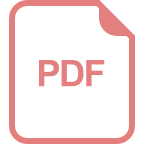

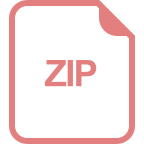
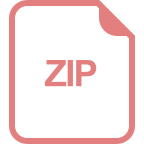
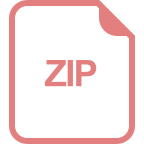
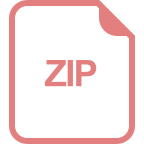
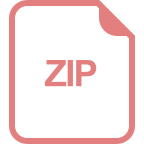
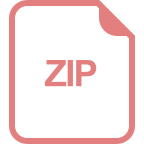
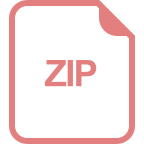
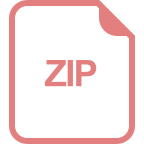
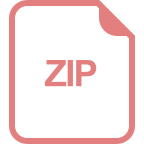
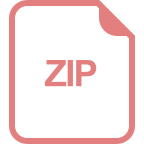