c++语言设计一个简单的走迷宫
时间: 2023-07-30 17:08:12 浏览: 58
好的,下面是一个基于C++的简单迷宫游戏实现,供您参考:
```c++
#include <iostream>
#include <vector>
#include <stack>
#include <cstdlib>
#include <ctime>
using namespace std;
// 定义迷宫元素类型
enum class MazeElement {
Wall = 0, // 墙壁
Path = 1, // 路径
Start = 2, // 起点
End = 3 // 终点
};
// 定义迷宫结构体
struct Maze {
int rows; // 迷宫行数
int cols; // 迷宫列数
int startX; // 起点横坐标
int startY; // 起点纵坐标
int endX; // 终点横坐标
int endY; // 终点纵坐标
vector<vector<MazeElement>> data; // 迷宫数据
};
// 随机生成迷宫
Maze generateMaze(int rows, int cols, int startX, int startY, int endX, int endY) {
Maze maze;
maze.rows = rows;
maze.cols = cols;
maze.startX = startX;
maze.startY = startY;
maze.endX = endX;
maze.endY = endY;
maze.data.resize(rows, vector<MazeElement>(cols, MazeElement::Wall));
// 随机生成迷宫
srand(time(nullptr));
stack<pair<int, int>> stk;
stk.push(make_pair(startX, startY));
maze.data[startX][startY] = MazeElement::Path;
while (!stk.empty()) {
auto curr = stk.top();
stk.pop();
int currX = curr.first, currY = curr.second;
vector<pair<int, int>> neighbors;
if (currX > 0 && maze.data[currX - 1][currY] == MazeElement::Wall) {
neighbors.push_back(make_pair(currX - 1, currY));
}
if (currX < rows - 1 && maze.data[currX + 1][currY] == MazeElement::Wall) {
neighbors.push_back(make_pair(currX + 1, currY));
}
if (currY > 0 && maze.data[currX][currY - 1] == MazeElement::Wall) {
neighbors.push_back(make_pair(currX, currY - 1));
}
if (currY < cols - 1 && maze.data[currX][currY + 1] == MazeElement::Wall) {
neighbors.push_back(make_pair(currX, currY + 1));
}
if (!neighbors.empty()) {
stk.push(curr);
int nextIndex = rand() % neighbors.size();
auto next = neighbors[nextIndex];
int nextX = next.first, nextY = next.second;
maze.data[nextX][nextY] = MazeElement::Path;
if (nextX == endX && nextY == endY) {
break;
}
stk.push(next);
}
}
maze.data[startX][startY] = MazeElement::Start;
maze.data[endX][endY] = MazeElement::End;
return maze;
}
// 打印迷宫
void printMaze(const Maze& maze) {
for (int i = 0; i < maze.rows; ++i) {
for (int j = 0; j < maze.cols; ++j) {
switch (maze.data[i][j]) {
case MazeElement::Wall:
cout << "# ";
break;
case MazeElement::Path:
cout << ". ";
break;
case MazeElement::Start:
cout << "S ";
break;
case MazeElement::End:
cout << "E ";
break;
}
}
cout << endl;
}
}
int main() {
// 随机生成一个迷宫
Maze maze = generateMaze(10, 10, 0, 0, 9, 9);
// 打印迷宫
printMaze(maze);
return 0;
}
```
以上代码实现了一个简单的迷宫游戏,随机生成一个10x10的迷宫,并打印出来。在实际游戏中,您可以根据上述代码实现更加完整的游戏逻辑和交互界面。
相关推荐
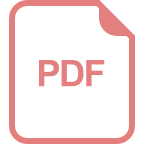
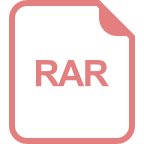
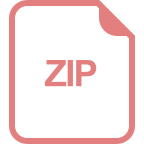
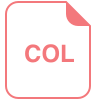
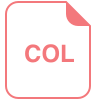





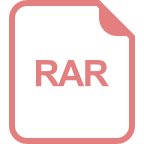
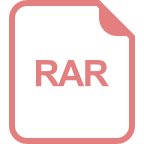
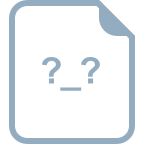
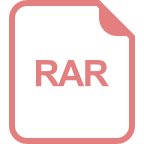
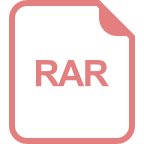
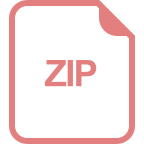
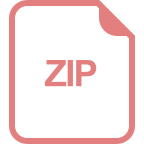