spring boot2 和spring boot 3 都是怎么连接数据库,具体代码是什么包括 pom.xml的配置是什么,分别是5.0以上数据库在ide里面要怎么配置和8.0以上的数据库也应该在哪里配置具体代码是什么
时间: 2024-09-18 13:00:33 浏览: 30
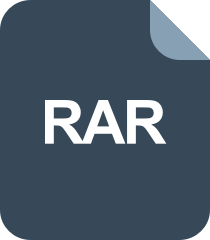
spring-tx-5.0.0.0.RELEASE.jar_java开发_spring-tx-5.0.0_
Spring Boot 2.x和Spring Boot 3.x(实际上Spring Boot 3尚未正式发布,这里假设的是使用Spring Boot 2.x作为例子)连接数据库的过程通常是通过依赖Spring Data JPA或者JDBC来完成的。以下是基本的配置步骤:
**Spring Boot 2.x 示例(使用Spring Data JPA)**:
1. **pom.xml配置**:
```xml
<dependencies>
<dependency>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter-data-jpa</artifactId>
</dependency>
<!-- 如果你的数据库是MySQL -->
<dependency>
<groupId>mysql</groupId>
<artifactId>mysql-connector-java</artifactId>
<scope>runtime</scope>
</dependency>
<!-- 如果是PostgreSQL -->
<dependency>
<groupId>org.postgresql</groupId>
<artifactId>postgresql</artifactId>
<scope>runtime</scope>
</dependency>
</dependencies>
```
2. **application.properties或application.yml文件** (假设数据库名为mydb, 数据库用户名和密码为user/pass):
```properties
spring.datasource.url=jdbc:mysql://localhost:3306/mydb?useSSL=false
spring.datasource.username=user
spring.datasource.password=pass
spring.jpa.hibernate.ddl-auto=update
```
3. **Repository接口示例** (MyEntity.java):
```java
import org.springframework.data.repository.CrudRepository;
public interface MyRepository extends CrudRepository<MyEntity, Long> {
}
```
**Spring Boot 2.x 示例(使用JDBC)**:
1. **pom.xml配置**:
```xml
<dependencies>
<dependency>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter-jdbc</artifactId>
</dependency>
<!-- 针对特定数据库的驱动 -->
<dependency>
<groupId>com.microsoft.sqlserver</groupId>
<artifactId>mssql-jdbc</artifactId> <!-- 使用SQL Server的驱动 -->
<scope>runtime</scope>
</dependency>
</dependencies>
```
2. **DataSource配置** (application.properties):
```properties
spring.datasource.url=jdbc:sqlserver://localhost:1433;databaseName=mydb
spring.datasource.username=user
spring.datasource.password=pass
```
3. **JdbcTemplate示例** (YourService.java):
```java
@Autowired
private JdbcTemplate jdbcTemplate;
...
List<MyEntity> entities = jdbcTemplate.query("SELECT * FROM MyTable", rs -> ...);
```
对于Spring Boot 8.0+(实际上可能是Spring Boot 3.0),配置方式大体上保持一致,只是可能会有一些新的特性引入,比如更多的自动配置选项。记得查看Spring官方文档以获取最新信息。
阅读全文
相关推荐
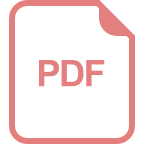
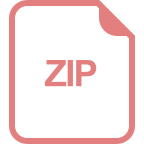
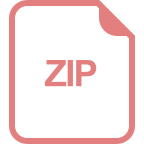
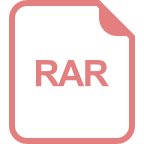
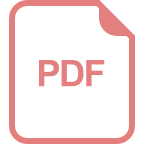
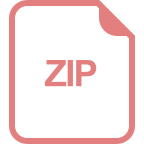
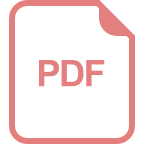
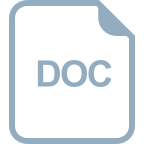
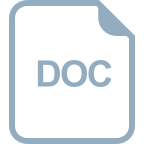
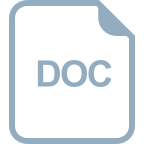
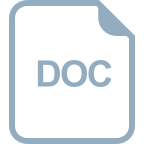
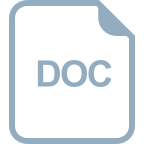
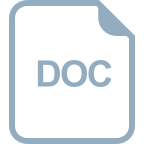
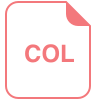
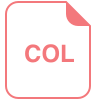
