用C语言 编制一个能演示执行集合的交、并和差运算的程序。 【任务要求】 1)集合元素用小写英文字母或者数字字符表示,执行各种操作应以对话方式执行。 2)算法要点:利用单链表表示集合;理解好三种运算的含义
时间: 2024-03-10 14:43:21 浏览: 67
好的,理解了您的要求。下面是一个简单的用 C 语言编写的程序,可以进行集合的交、并和差运算。程序采用单链表实现集合,具体实现如下:
```c
#include <stdio.h>
#include <stdlib.h>
#include <string.h>
// 定义集合中元素的最大长度
#define MAX_LEN 50
// 定义集合元素的结构体
typedef struct Element {
char value[MAX_LEN];
struct Element *next;
} Element;
// 定义集合的结构体
typedef struct Set {
Element *head;
int size;
} Set;
// 初始化集合
Set* initSet() {
Set *s = (Set *)malloc(sizeof(Set));
s->head = NULL;
s->size = 0;
return s;
}
// 判断集合是否为空
int isEmpty(Set *s) {
return s->size == 0;
}
// 判断元素是否在集合中
int isInSet(Set *s, char *value) {
Element *p = s->head;
while (p != NULL) {
if (strcmp(p->value, value) == 0) {
return 1;
}
p = p->next;
}
return 0;
}
// 向集合中添加元素
void addElement(Set *s, char *value) {
if (isInSet(s, value)) {
printf("%s already exists in the set.\n", value);
return;
}
Element *e = (Element *)malloc(sizeof(Element));
strcpy(e->value, value);
e->next = s->head;
s->head = e;
s->size++;
}
// 从集合中删除元素
void removeElement(Set *s, char *value) {
if (isEmpty(s)) {
printf("The set is empty.\n");
return;
}
Element *p = s->head, *pre = NULL;
while (p != NULL) {
if (strcmp(p->value, value) == 0) {
if (pre == NULL) {
s->head = p->next;
} else {
pre->next = p->next;
}
free(p);
s->size--;
printf("%s has been removed from the set.\n", value);
return;
}
pre = p;
p = p->next;
}
printf("%s is not in the set.\n", value);
}
// 打印集合中的元素
void printSet(Set *s) {
if (isEmpty(s)) {
printf("The set is empty.\n");
return;
}
printf("Set: { ");
Element *p = s->head;
while (p != NULL) {
printf("%s ", p->value);
p = p->next;
}
printf("}\n");
}
// 释放集合中的内存空间
void freeSet(Set *s) {
Element *p = s->head, *pre = NULL;
while (p != NULL) {
pre = p;
p = p->next;
free(pre);
}
free(s);
}
// 求集合的交集
Set* intersection(Set *s1, Set *s2) {
Set *result = initSet();
Element *p = s1->head;
while (p != NULL) {
if (isInSet(s2, p->value)) {
addElement(result, p->value);
}
p = p->next;
}
return result;
}
// 求集合的并集
Set* unionSet(Set *s1, Set *s2) {
Set *result = initSet();
Element *p = s1->head;
while (p != NULL) {
addElement(result, p->value);
p = p->next;
}
p = s2->head;
while (p != NULL) {
addElement(result, p->value);
p = p->next;
}
return result;
}
// 求集合的差集
Set* difference(Set *s1, Set *s2) {
Set *result = initSet();
Element *p = s1->head;
while (p != NULL) {
if (!isInSet(s2, p->value)) {
addElement(result, p->value);
}
p = p->next;
}
return result;
}
int main() {
Set *s1 = initSet();
addElement(s1, "a");
addElement(s1, "b");
addElement(s1, "c");
printf("Set 1: ");
printSet(s1);
Set *s2 = initSet();
addElement(s2, "b");
addElement(s2, "c");
addElement(s2, "d");
printf("Set 2: ");
printSet(s2);
Set *s3 = intersection(s1, s2);
printf("Intersection of Set 1 and Set 2: ");
printSet(s3);
freeSet(s3);
Set *s4 = unionSet(s1, s2);
printf("Union of Set 1 and Set 2: ");
printSet(s4);
freeSet(s4);
Set *s5 = difference(s1, s2);
printf("Difference of Set 1 and Set 2: ");
printSet(s5);
freeSet(s5);
removeElement(s1, "a");
printf("Set 1 after removing element 'a': ");
printSet(s1);
freeSet(s1);
freeSet(s2);
return 0;
}
```
在程序中,我们定义了集合元素和集合的结构体,使用单链表实现了集合的基本操作,包括:
- 初始化集合
- 判断集合是否为空
- 判断元素是否在集合中
- 向集合中添加元素
- 从集合中删除元素
- 打印集合中的元素
- 释放集合中的内存空间
然后我们实现了三种集合运算:
- 求集合的交集 `intersection`
- 求集合的并集 `unionSet`
- 求集合的差集 `difference`
最后,在 `main` 函数中我们演示了如何使用这些函数来进行集合运算。
阅读全文
相关推荐
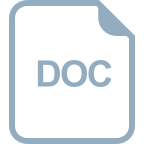
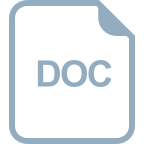
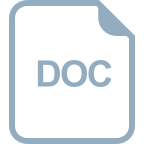











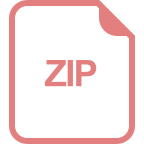
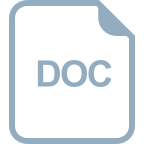
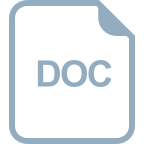
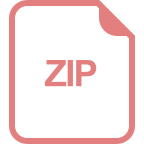