nodejs 读取本地文件转成file对象
时间: 2024-01-31 16:03:23 浏览: 464
在Node.js环境下,可以使用`fs`模块读取本地文件,并通过`Blob`对象将文件内容转为`File`对象。以下是一个示例代码:
```javascript
const fs = require('fs');
const { Blob } = require('buffer');
const filePath = '/path/to/file.txt';
const fileContent = fs.readFileSync(filePath);
const file = new File([new Blob([fileContent])], 'file.txt');
```
其中,`fs.readFileSync`方法用于同步读取文件内容,返回一个`Buffer`对象。然后通过`Blob`对象将`Buffer`对象转为二进制数据,再将其传入`File`对象的构造函数中即可。
需要注意的是,`Blob`对象需要通过`buffer`模块引入,而不是通过`blob`模块引入,因为在Node.js环境下,`blob`模块不支持。
相关问题
electron nodejs读取本地视频文件通过video打开
要使用Electron和Node.js在浏览器中打开本地视频,你可以使用Node.js的`fs`模块来读取本地视频文件,然后将文件路径作为`<video>`标签的`src`属性值,最后使用Electron的`BrowserWindow`模块来创建一个包含`<video>`标签的窗口。
具体步骤如下:
1. 在你的Electron项目目录下,创建一个视频文件夹,并将你的本地视频文件放入该文件夹中。
2. 在你的JavaScript文件中,使用`fs`模块读取本地视频文件,示例代码如下:
```javascript
const { app, BrowserWindow } = require('electron');
const path = require('path');
const url = require('url');
const fs = require('fs');
let mainWindow;
function createWindow() {
mainWindow = new BrowserWindow({
width: 800,
height: 600,
webPreferences: {
nodeIntegration: true,
},
});
const filePath = path.join(__dirname, '/videos/video.mp4'); // 视频文件路径
const fileExists = fs.existsSync(filePath);
if (fileExists) {
mainWindow.loadURL(url.format({
pathname: path.join(__dirname, 'index.html'),
protocol: 'file:',
slashes: true,
}));
mainWindow.webContents.on('did-finish-load', () => {
mainWindow.webContents.send('play-video', filePath);
});
} else {
console.error('视频文件不存在');
}
mainWindow.on('closed', () => {
mainWindow = null;
});
}
app.on('ready', createWindow);
app.on('window-all-closed', () => {
if (process.platform !== 'darwin') {
app.quit();
}
});
app.on('activate', () => {
if (mainWindow === null) {
createWindow();
}
});
```
其中,`filePath`是你本地视频文件的路径,`fileExists`变量用于判断视频文件是否存在。
3. 在你的HTML文件中,创建一个包含`<video>`标签的页面,示例代码如下:
```html
<!DOCTYPE html>
<html>
<head>
<meta charset="UTF-8">
<title>My Video Player</title>
</head>
<body>
<video id="video-player" width="640" height="360" controls>
Your browser does not support the video tag.
</video>
<script src="./renderer.js"></script>
</body>
</html>
```
其中,`<video>`标签的`id`属性为`video-player`,`controls`属性用于显示视频控制条。
4. 在你的renderer.js文件中,使用`ipcRenderer`模块来接收主进程发送的视频文件路径,并将其作为`<video>`标签的`src`属性值,示例代码如下:
```javascript
const { ipcRenderer } = require('electron');
ipcRenderer.on('play-video', (event, filePath) => {
const videoPlayer = document.getElementById('video-player');
videoPlayer.src = `file://${filePath}`;
});
```
其中,`ipcRenderer.on('play-video', ...)`用于接收主进程发送的视频文件路径,`videoPlayer.src`用于将其作为`<video>`标签的`src`属性值。
5. 运行JavaScript文件,即可通过Electron打开包含`<video>`标签的窗口,自动播放指定的本地视频文件。
nodejs 读取本地服务器图片
Node.js 本身是一个运行在服务端的 JavaScript 环境,它并不直接支持像浏览器那样访问本地文件系统。但是,如果你是在开发过程中需要处理本地图片文件,通常会借助一些 Node.js 的模块或者中间件来实现。
一种常见的做法是使用 `fs` 模块(File System)来读取本地文件,然后将图片内容转换为可以发送到客户端的数据流。例如,你可以这样做:
```javascript
const fs = require('fs');
const path = require('path');
// 定义图片文件路径
const imagePath = path.join(__dirname, 'your_image.jpg');
async function readImage() {
try {
const fileStream = fs.createReadStream(imagePath);
// 将文件流转化为 Buffer 或者 Base64 字符串,取决于需求
const imageBuffer = await new Promise((resolve) => {
fileStream.on('data', (chunk) => resolve(chunk));
fileStream.on('end', () => resolve(null));
});
if (imageBuffer) {
// 发送给客户端或者其他地方
console.log('Image buffer:', imageBuffer.toString('base64')); // 如果是 base64 编码
}
} catch (err) {
console.error('Error reading image:', err);
}
}
readImage();
```
阅读全文
相关推荐
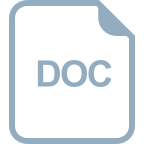
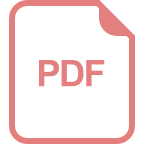



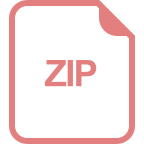
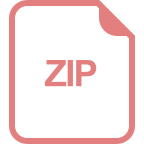
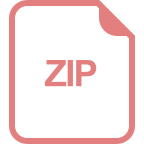







