nodejs读写json文件的简单方法
时间: 2023-09-08 10:02:28 浏览: 50
在Node.js中,读写JSON文件有多种简单方法可以实现。
读取JSON文件:
1. 使用内置的fs模块的readFileSync函数来同步地读取JSON文件内容,并使用JSON.parse将其转换为对象或数组:
```javascript
const fs = require('fs');
try {
const data = fs.readFileSync('data.json', 'utf8');
const jsonData = JSON.parse(data);
console.log(jsonData);
} catch (error) {
console.error(error);
}
```
2. 使用fs模块的readFile函数来异步读取JSON文件内容,并在回调函数中处理读取的数据:
```javascript
const fs = require('fs');
fs.readFile('data.json', 'utf8', (error, data) => {
if (error) {
console.error(error);
} else {
const jsonData = JSON.parse(data);
console.log(jsonData);
}
});
```
写入JSON文件:
1. 使用fs模块的writeFileSync函数同步地写入JSON数据到文件中:
```javascript
const fs = require('fs');
const jsonData = {
name: 'John Doe',
age: 30,
email: 'johndoe@example.com'
};
try {
fs.writeFileSync('data.json', JSON.stringify(jsonData, null, 2));
console.log('JSON data has been written to file.');
} catch (error) {
console.error(error);
}
```
2. 使用fs模块的writeFile函数异步地写入JSON数据到文件中:
```javascript
const fs = require('fs');
const jsonData = {
name: 'John Doe',
age: 30,
email: 'johndoe@example.com'
};
fs.writeFile('data.json', JSON.stringify(jsonData, null, 2), (error) => {
if (error) {
console.error(error);
} else {
console.log('JSON data has been written to file.');
}
});
```
以上是node.js读写JSON文件的简单方法。可以根据实际需求选择适合的方法。
相关推荐
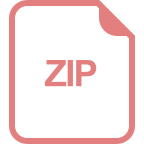
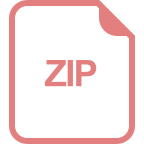









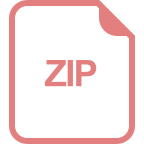
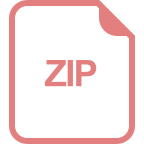
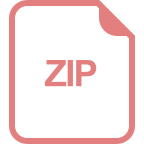
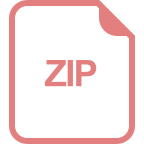
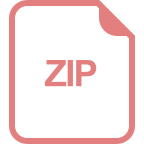