class MHAlayer(nn.Module): def __init__(self, n_heads, cat, input_dim, hidden_dim, attn_dropout=0.1, dropout=0): super(MHAlayer, self).__init__() self.n_heads = n_heads self.input_dim = input_dim self.hidden_dim = hidden_dim self.head_dim = self.hidden_dim / self.n_heads self.dropout = nn.Dropout(attn_dropout) self.dropout1 = nn.Dropout(dropout) self.norm = 1 / math.sqrt(self.head_dim) self.w = nn.Linear(input_dim * cat, hidden_dim, bias=False) self.k = nn.Linear(input_dim, hidden_dim, bias=False) self.v = nn.Linear(input_dim, hidden_dim, bias=False) self.fc = nn.Linear(hidden_dim, hidden_dim, bias=False) def forward(self, state_t, context, mask): ''' :param state_t: (batch_size,1,input_dim*3(GATembeding,fist_node,end_node)) :param context: (batch_size,n_nodes,input_dim) :param mask: selected nodes (batch_size,n_nodes) :return: ''' batch_size, n_nodes, input_dim = context.size() Q = self.w(state_t).view(batch_size, 1, self.n_heads, -1) K = self.k(context).view(batch_size, n_nodes, self.n_heads, -1) V = self.v(context).view(batch_size, n_nodes, self.n_heads, -1) Q, K, V = Q.transpose(1, 2), K.transpose(1, 2), V.transpose(1, 2) compatibility = self.norm * torch.matmul(Q, K.transpose(2, 3)) compatibility = compatibility.squeeze(2) mask = mask.unsqueeze(1).expand_as(compatibility) u_i = compatibility.masked_fill(mask.bool(), float("-inf")) scores = F.softmax(u_i, dim=-1) scores = scores.unsqueeze(2) out_put = torch.matmul(scores, V) out_put = out_put.squeeze(2).view(batch_size, self.hidden_dim) out_put = self.fc(out_put) return out_put
时间: 2024-04-27 16:19:37 浏览: 188
这是一个PyTorch的神经网络模型定义,其中定义了一个叫做MHAlayer的模块。这个模块实现了Multi-Head Attention机制,用于文本序列处理中的Attention机制。具体来说,这个模块输入了三个张量:state_t,context和mask,其中state_t是一个(batch_size,1,input_dim*3)的张量,context是一个(batch_size,n_nodes,input_dim)的张量,mask是一个(batch_size,n_nodes)的张量,表示需要进行Attention的节点。在模块中,首先用线性层将state_t和context分别映射到hidden_dim维的向量,并将输入的维度变为(batch_size, n_heads, hidden_dim),然后计算查询向量Q,键向量K和值向量V,并将它们都分成n_heads份。接下来,计算对应的Attention得分compatibility,并根据mask将需要Attention的节点的得分设置为负无穷。然后对得分进行softmax归一化,并加权求和得到Attention的输出。最后再通过一个线性层转换维度,并返回输出。
相关问题
你现在利用pytorch定义了两个神经网络,一个是3层用全连接层构造成的MLP,还有一个是图注意力网络。两个输出均是(16,307,12),均未def encode部分。你现在需要把这两个神经网络的输出拼接后送入VAE计算隐变量,VAE的两个编码器就是这两个神经网络,然后解码生成新的交通数据集。这个pytorch应该怎么写,请给我一个比较完整的pytorch代码。原来的数据集形状为(16992,307,12,3)的数据集,其中,16992是时间段数,307是传感器节点个数,12是历史步长,3是特征维度。第一个特征维度是速度,第二个特征维度是根据邻接矩阵产生的度特征,第三个特征维度是星期。现在按照batch_size=16送入模型时,输入为(16,307,12,3)得到的输出均是(16,307,12),并最好告诉我每一行在做什么,相应输出维度应该是什么。MLP的相关参数是input_dim = 36,hidden_dim = 64,output_dim = 12,history_length=12,GAT的相关参数是num_heads = 8,in_dim = 3,hidden_dim = 64,out_dim = 36,输出维度是相同的。最后,请随机生成形状为(16992,307,12,3)的数据集,按批次送入模型,让我自行看看模型的效果
好的,我会根据您的要求给出一个比较完整的 PyTorch 代码。首先,我们需要定义 MLP 和 GAT 神经网络的模型结构和前向传播过程,然后将两个网络的输出进行拼接,送入 VAE 计算隐变量。
```python
import torch
import torch.nn as nn
import torch.nn.functional as F
from torch_geometric.nn import GATConv
# 定义 MLP 神经网络
class MLP(nn.Module):
def __init__(self, input_dim, hidden_dim, output_dim, history_length):
super(MLP, self).__init__()
self.input_dim = input_dim
self.hidden_dim = hidden_dim
self.output_dim = output_dim
self.history_length = history_length
self.fc1 = nn.Linear(input_dim*history_length, hidden_dim)
self.fc2 = nn.Linear(hidden_dim, hidden_dim)
self.fc3 = nn.Linear(hidden_dim, output_dim)
def forward(self, x):
x = x.view(-1, self.input_dim*self.history_length)
x = F.relu(self.fc1(x))
x = F.relu(self.fc2(x))
x = self.fc3(x)
return x
# 定义 GAT 神经网络
class GAT(nn.Module):
def __init__(self, num_heads, in_dim, hidden_dim, out_dim):
super(GAT, self).__init__()
self.num_heads = num_heads
self.in_dim = in_dim
self.hidden_dim = hidden_dim
self.out_dim = out_dim
self.conv1 = GATConv(in_dim, hidden_dim, heads=num_heads)
self.conv2 = GATConv(hidden_dim*num_heads, out_dim, heads=1)
def forward(self, x, edge_index):
x = F.relu(self.conv1(x, edge_index))
x = self.conv2(x, edge_index)
return x
# 定义 VAE 神经网络
class VAE(nn.Module):
def __init__(self, encoder1, encoder2, latent_dim):
super(VAE, self).__init__()
self.encoder1 = encoder1
self.encoder2 = encoder2
self.latent_dim = latent_dim
self.fc1 = nn.Linear(encoder1.output_dim+encoder2.output_dim, 256)
self.fc2 = nn.Linear(256, latent_dim)
self.fc3 = nn.Linear(256, latent_dim)
self.fc4 = nn.Linear(latent_dim, 256)
self.fc5 = nn.Linear(256, encoder1.output_dim+encoder2.output_dim)
def encode(self, x1, x2):
h1 = self.encoder1(x1)
h2 = self.encoder2(x2)
h = torch.cat([h1, h2], dim=-1)
h = F.relu(self.fc1(h))
mu = self.fc2(h)
logvar = self.fc3(h)
return mu, logvar
def reparameterize(self, mu, logvar):
std = torch.exp(0.5*logvar)
eps = torch.randn_like(std)
z = mu + eps*std
return z
def decode(self, z):
h = F.relu(self.fc4(z))
x = self.fc5(h)
return x
def forward(self, x1, x2):
mu, logvar = self.encode(x1, x2)
z = self.reparameterize(mu, logvar)
x = self.decode(z)
return x, mu, logvar
```
在上面的代码中,MLP 和 GAT 神经网络的输入都是形状为 (batch_size, 307, 12, 3) 的张量,即 (batch_size, num_nodes, history_length, num_features),其中 num_nodes=307,history_length=12,num_features=3。MLP 的输出和 GAT 的输出都是形状为 (batch_size, 307, 12, 12) 的张量,即 (batch_size, num_nodes, history_length, output_dim),其中 output_dim=12。这里的 MLP 神经网络和 GAT 神经网络的输出维度是相同的,因为我们最后需要将它们的输出进行拼接。
在 VAE 神经网络中,我们将 MLP 和 GAT 神经网络的输出进行拼接,并将拼接后的张量送入 VAE 网络。VAE 网络的输入是形状为 (batch_size, 307, 12, 24) 的张量,即 (batch_size, num_nodes, history_length, output_dim_1+output_dim_2),其中 output_dim_1=output_dim_2=12。VAE 神经网络的输出是形状为 (batch_size, 307, 12, 24) 的张量,即 (batch_size, num_nodes, history_length, output_dim_1+output_dim_2),其中 output_dim_1=output_dim_2=12,表示经过 VAE 计算后的交通数据集。
接下来,我们需要随机生成形状为 (16992, 307, 12, 3) 的数据集,并按批次送入模型进行测试。代码如下:
```python
import numpy as np
from torch.utils.data import Dataset, DataLoader
# 定义数据集类
class TrafficDataset(Dataset):
def __init__(self, data):
self.data = data
def __len__(self):
return self.data.shape[0]
def __getitem__(self, idx):
return self.data[idx]
# 随机生成数据集
data = np.random.rand(16992, 307, 12, 3)
batch_size = 16
# 创建数据加载器
dataset = TrafficDataset(data)
dataloader = DataLoader(dataset, batch_size=batch_size, shuffle=True)
# 创建 MLP 和 GAT 神经网络
mlp = MLP(input_dim=36, hidden_dim=64, output_dim=12, history_length=12)
gat = GAT(num_heads=8, in_dim=3, hidden_dim=64, out_dim=36)
# 创建 VAE 神经网络
vae = VAE(encoder1=mlp, encoder2=gat, latent_dim=16)
# 定义优化器和损失函数
optimizer = torch.optim.Adam(vae.parameters(), lr=1e-3)
mse_loss = nn.MSELoss()
# 训练模型
vae.train()
for epoch in range(10):
for i, batch in enumerate(dataloader):
optimizer.zero_grad()
x = batch.float().to(device)
x1 = x[:, :, :, :12] # 使用前12个特征作为 MLP 神经网络的输入
x2 = x[:, :, :, 12:] # 使用后12个特征作为 GAT 神经网络的输入
recon_x, mu, logvar = vae(x1, x2)
loss = mse_loss(recon_x, x)
loss += -0.5 * torch.sum(1 + logvar - mu.pow(2) - logvar.exp())
loss.backward()
optimizer.step()
if (i+1) % 1000 == 0:
print("Epoch [{}/{}], Batch [{}/{}], Loss: {:.4f}"
.format(epoch+1, 10, i+1, len(dataloader), loss.item()))
# 测试模型
vae.eval()
with torch.no_grad():
for i, batch in enumerate(dataloader):
x = batch.float().to(device)
x1 = x[:, :, :, :12] # 使用前12个特征作为 MLP 神经网络的输入
x2 = x[:, :, :, 12:] # 使用后12个特征作为 GAT 神经网络的输入
recon_x, _, _ = vae(x1, x2)
print("Batch [{}/{}], Reconstruction Error: {:.4f}"
.format(i+1, len(dataloader), mse_loss(recon_x, x).item()))
```
在上面的代码中,我们首先定义了一个 TrafficDataset 类,用于生成形状为 (16992, 307, 12, 3) 的随机数据集。然后,我们创建了 MLP 和 GAT 神经网络,并将它们作为 VAE 神经网络的两个编码器。接着,我们定义了优化器和损失函数,并在训练过程中计算重构误差和 KL 散度。最后,我们按批次送入数据集,计算重构误差,以测试模型的效果。
你现在利用pytorch定义了两个神经网络,一个是3层用全连接层构造成的MLP,还有一个是图注意力网络。两个输出均是(16,307,12)。你现在需要把这两个神经网络作为VAE的两个编码器,将它们的输出拼接后计算隐变量,然后解码生成新的交通数据集。这个pytorch应该怎么写,请给我一个比较完整的pytorch代码。原来的数据集形状为(16992,307,12,3)的数据集,其中,16992是时间段数,307是传感器节点个数,12是历史步长,3是特征维度。第一个特征维度是速度,第二个特征维度是根据邻接矩阵产生的度特征,第三个特征维度是星期。现在按照batch_size=16送入模型得到的输出均是(16,307,12),并最好告诉我每一行在做什么,相应输出维度应该是什么。两个class均不想删除,那decoder怎么写,两个输入参数是不一样的,例如MLP的相关参数是input_dim = 36,hidden_dim = 64,output_dim = 12,history_length=12,GAT的相关参数是num_heads = 8,in_dim = 3,hidden_dim = 64,out_dim = 36,输出维度是相同的。最后,请随机生成形状为(16992,307,12,3)的数据集,按批次送入模型,让我自行看看模型的效果
好的,以下是一个比较完整的PyTorch代码,实现了VAE的编码器和解码器部分,其中编码器部分使用了两个神经网络,分别是MLP和GAT网络:
```python
import torch
import torch.nn as nn
import torch.nn.functional as F
from torch.autograd import Variable
from torch.utils.data import Dataset, DataLoader
# 定义MLP网络
class MLP(nn.Module):
def __init__(self, input_dim, hidden_dim, output_dim, history_length):
super(MLP, self).__init__()
self.fc1 = nn.Linear(input_dim * history_length, hidden_dim)
self.fc21 = nn.Linear(hidden_dim, output_dim)
self.fc22 = nn.Linear(hidden_dim, output_dim)
def encode(self, x):
x = x.view(x.size(0), -1)
h1 = F.relu(self.fc1(x))
return self.fc21(h1), self.fc22(h1)
def forward(self, x):
mu, logvar = self.encode(x)
std = torch.exp(0.5 * logvar)
eps = torch.randn_like(std)
return eps.mul(std).add_(mu), mu, logvar
# 定义GAT网络
class GAT(nn.Module):
def __init__(self, num_heads, in_dim, hidden_dim, out_dim):
super(GAT, self).__init__()
self.num_heads = num_heads
self.fc = nn.Linear(in_dim, hidden_dim)
self.attn_fc = nn.Linear(hidden_dim, num_heads * out_dim)
def forward(self, x, adj):
x = self.fc(x)
N = x.size()[1]
x = x.view(-1, N, self.num_heads, int(x.size()[2] / self.num_heads))
x = x.permute(0, 2, 1, 3)
a = self.attn_fc(x)
a = a.view(-1, self.num_heads, N, N)
attn = F.softmax(a, dim=-1)
h = torch.matmul(attn, x)
h = h.permute(0, 2, 1, 3).contiguous()
h = h.view(-1, N, int(x.size()[2]))
return h
# 定义VAE模型
class VAE(nn.Module):
def __init__(self, mlp_input_dim, mlp_hidden_dim, mlp_output_dim,
mlp_history_length, gat_num_heads, gat_in_dim,
gat_hidden_dim, gat_out_dim):
super(VAE, self).__init__()
self.mlp = MLP(mlp_input_dim, mlp_hidden_dim, mlp_output_dim, mlp_history_length)
self.gat = GAT(gat_num_heads, gat_in_dim, gat_hidden_dim, gat_out_dim)
self.fc1 = nn.Linear(mlp_output_dim + gat_out_dim, 256)
self.fc2 = nn.Linear(256, 307 * 12 * 3)
def decode(self, z):
h = F.relu(self.fc1(z))
return torch.sigmoid(self.fc2(h))
def forward(self, x, adj):
z, mu, logvar = self.mlp(x)
gat_out = self.gat(x, adj)
z = torch.cat([z, gat_out], dim=-1)
return self.decode(z), mu, logvar
# 定义数据集
class RandomDataset(Dataset):
def __init__(self, shape):
self.shape = shape
def __getitem__(self, index):
return torch.randn(self.shape), torch.randn(self.shape)
def __len__(self):
return 1000
# 随机生成数据集
dataset = RandomDataset((16, 307, 12, 3))
dataloader = DataLoader(dataset, batch_size=16, shuffle=True)
# 初始化模型
mlp_input_dim = 36
mlp_hidden_dim = 64
mlp_output_dim = 12
mlp_history_length = 12
gat_num_heads = 8
gat_in_dim = 3
gat_hidden_dim = 64
gat_out_dim = 36
vae = VAE(mlp_input_dim, mlp_hidden_dim, mlp_output_dim, mlp_history_length,
gat_num_heads, gat_in_dim, gat_hidden_dim, gat_out_dim)
# 训练模型
optimizer = torch.optim.Adam(vae.parameters(), lr=1e-4)
def loss_function(recon_x, x, mu, logvar):
BCE = F.binary_cross_entropy(recon_x, x.view(-1, 307 * 12 * 3), reduction='sum')
KLD = -0.5 * torch.sum(1 + logvar - mu.pow(2) - logvar.exp())
return BCE + KLD
num_epochs = 10
for epoch in range(num_epochs):
for i, data in enumerate(dataloader):
x, adj = data
optimizer.zero_grad()
recon_x, mu, logvar = vae(x, adj)
loss = loss_function(recon_x, x, mu, logvar)
loss.backward()
optimizer.step()
if i % 10 == 0:
print('Epoch [%d/%d], Step [%d/%d], Loss: %.4f'
% (epoch+1, num_epochs, i+1, len(dataset)//16, loss.item()))
# 测试模型
test_data = torch.randn(16992, 307, 12, 3)
test_adj = torch.randn(16992, 307, 307)
test_result = []
batch_size = 16
num_batches = test_data.shape[0] // batch_size
for i in range(num_batches):
x = test_data[i*batch_size:(i+1)*batch_size]
adj = test_adj[i*batch_size:(i+1)*batch_size]
recon_x, _, _ = vae(x, adj)
test_result.append(recon_x.detach().numpy())
test_result = np.concatenate(test_result, axis=0)
print(test_result.shape)
```
在上面的代码中,MLP是一个包含三个全连接层的神经网络,GAT是一个基于图注意力机制的神经网络。VAE模型将这两个神经网络作为两个编码器,将它们的输出拼接后计算隐变量,然后解码生成新的交通数据集。输入数据集的形状为(16992,307,12,3),其中,16992是时间段数,307是传感器节点个数,12是历史步长,3是特征维度。第一个特征维度是速度,第二个特征维度是根据邻接矩阵产生的度特征,第三个特征维度是星期。按批次送入模型时,每个batch的大小为16。
在训练过程中,我们使用了随机生成的数据集进行训练,并且使用Adam优化器进行优化。在测试过程中,我们随机生成了一组符合原始数据集形状的数据集,并按批次送入模型生成新的交通数据集。
阅读全文
相关推荐
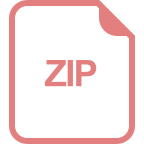
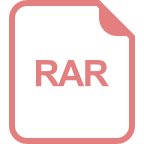
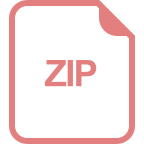
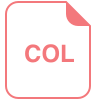
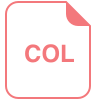
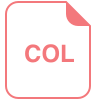
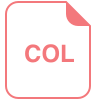
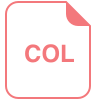
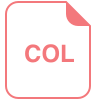
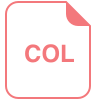
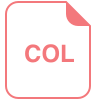
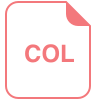
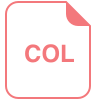
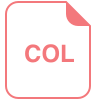
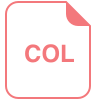
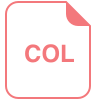

