C++ try catch std::cerr
时间: 2023-10-21 07:28:37 浏览: 55
您好!对于您的问题,我会使用 C++ 的 try-catch 块来捕获异常,并使用 std::cerr 输出错误信息。下面是一个简单的示例:
```cpp
#include <iostream>
#include <stdexcept>
int main() {
try {
// 在这里放置可能引发异常的代码
throw std::runtime_error("This is an example exception.");
}
catch (const std::exception& e) {
std::cerr << "Exception caught: " << e.what() << std::endl;
}
return 0;
}
```
在上面的示例中,我们使用 `std::runtime_error` 异常类抛出一个自定义错误消息。catch 块使用 `const std::exception&` 引用来捕获任何继承自 `std::exception` 的异常,并使用 `std::cerr` 输出错误消息。
请注意,这只是一个简单的示例,您可以根据自己的需求进行修改和扩展。
相关问题
C++ WORD转std::string
在C++中,将一个WORD文档转换为std::string可以通过使用适当的库来实现。一个常用的库是Microsoft Office的COM组件,可以使用它来操作WORD文档并将其内容转换为std::string。
以下是一个简单的示例代码,演示了如何使用COM组件将WORD文档转换为std::string:
```cpp
#include <iostream>
#include <string>
#include <comutil.h>
#include <comdef.h>
#include <atlbase.h>
std::string WordToStdString(const std::wstring& filePath)
{
std::string result;
// 初始化COM组件
CoInitialize(NULL);
try {
// 创建Word应用程序对象
_ApplicationPtr pWordApp;
HRESULT hr = pWordApp.CreateInstance(__uuidof(Application));
if (FAILED(hr)) {
throw std::runtime_error("Failed to create Word application object");
}
// 打开文档
DocumentsPtr pDocs = pWordApp->Documents;
_DocumentPtr pDoc = pDocs->Open(filePath.c_str());
// 提取文档内容
_bstr_t content = pDoc->Content->Text;
// 将内容转换为std::string
result = static_cast<const char*>(content);
// 关闭文档
pDoc->Close();
// 退出Word应用程序
pWordApp->Quit();
}
catch (const _com_error& e) {
std::cerr << "COM Error: " << e.ErrorMessage() << std::endl;
}
// 释放COM资源
CoUninitialize();
return result;
}
int main()
{
std::wstring filePath = L"example.docx";
std::string content = WordToStdString(filePath);
std::cout << "Content: " << content << std::endl;
return 0;
}
```
请注意,上述示例代码使用了`_ApplicationPtr`和`_DocumentPtr`等COM组件的智能指针,这些指针会自动处理COM资源的释放,避免内存泄漏。
c++ std::invalid_argument
### 回答1:
c std::invalid_argument是C++标准库中的一个异常类,用于表示参数无效的情况。
在C++编程中,当我们使用函数或方法时,需要给它传递一些参数。有时候,在函数执行之前,需要对这些参数进行一些有效性检查,以确保程序的正确性。
如果参数经过检查发现无效,也就是不符合预期的条件或范围,就可以抛出std::invalid_argument异常。这个异常是从std::logic_error类派生而来的,表示逻辑错误。
使用std::invalid_argument异常的时机可以是在函数内部进行参数检查,当发现参数无效时,通过throw语句抛出异常,并指定一个错误的描述信息。在函数的调用处,可以使用try-catch块进行异常处理,捕获并处理这个异常。
比如,我们可以写一个函数来计算一个正整数的平方根,当输入为负数时,我们可以认为这是一个无效的参数,可以抛出std::invalid_argument异常:
```cpp
#include <stdexcept>
#include <cmath>
double calculateSquareRoot(int num) {
if (num < 0) {
throw std::invalid_argument("输入的数值不能是负数!");
}
return sqrt(num);
}
int main() {
try {
double result = calculateSquareRoot(-1);
// 这里不会被执行,因为异常已经被抛出
std::cout << "结果是:" << result << std::endl;
} catch (const std::invalid_argument& e) {
std::cout << "发生了异常:" << e.what() << std::endl;
}
return 0;
}
```
在这个例子中,当我们调用calculateSquareRoot函数时,参数为-1,这是一个无效的参数。函数内部进行了检查,并抛出了std::invalid_argument异常。在main函数中,我们用try-catch块捕获并处理了这个异常,输出了错误信息"输入的数值不能是负数!"。
这样,使用std::invalid_argument异常可以帮助我们在编程中及时发现不符合预期的参数,并进行必要的处理。
### 回答2:
c std::invalid_argument是C++标准库中的一个异常类型。当函数接收到一个不合法的参数时,就会抛出该异常。
c std::invalid_argument是std命名空间下的一个类,用于表示无效参数的异常。当调用函数时,传入了无效的参数,例如传入一个超出允许范围的数值,或者传入一个格式不正确的字符串,函数就会抛出std::invalid_argument异常来提示调用者参数是无效的。
在处理该异常时,可以使用try-catch语句来捕获异常,然后进行相应的处理。catch块中可以输出错误信息,或者进行其他相关操作,比如使用默认值代替无效参数,或者向用户显示错误提示信息,以便修复无效的输入。
下面是一个简单的示例代码,展示了如何使用std::invalid_argument异常:
```cpp
#include <iostream>
#include <stdexcept>
int main() {
try {
int age;
std::cout << "请输入年龄:" << std::endl;
std::cin >> age;
if (age < 0 || age > 120) {
throw std::invalid_argument("年龄无效!");
}
std::cout << "年龄是:" << age << std::endl;
} catch(const std::invalid_argument& e) {
std::cerr << "捕获到异常:" << e.what() << std::endl;
}
return 0;
}
```
以上代码中,我们通过std::cin读取用户输入的年龄,然后判断是否是有效的年龄范围。如果不是,就通过throw语句抛出std::invalid_argument异常并附加错误信息"年龄无效!"。在catch块中,通过调用e.what()可以获取异常的错误信息并输出。
总之,c std::invalid_argument是用于表示无效参数的异常类型,可以帮助我们及时发现并处理调用函数时传入的无效参数。
### 回答3:
c std::invalid_argument是标准C++库中的一个异常类。这个异常类主要用于表示当一个函数接收到一个无效的参数时抛出的异常。
在C++中,异常是一种错误处理机制,用于在程序运行过程中发生错误时能够进行异常处理而不会导致程序崩溃。std::invalid_argument是C++标准库中的一个具体异常类,用于表示由无效参数引起的异常。
当一个函数接收到一个无效参数时,可以抛出std::invalid_argument异常。例如,如果一个函数期望接收一个正整数作为参数,但接收到了一个负数或者零,那么就可以抛出std::invalid_argument异常来表示参数无效。
使用std::invalid_argument异常可以在函数内部进行错误处理,还可以将异常传递给调用该函数的代码块进行处理。通过捕获这个异常,我们可以针对具体的无效参数情况进行处理,比如输出错误信息或者进行其他逻辑处理。
总之,C++中的std::invalid_argument异常是用于表示函数接收到无效参数时抛出的异常类,它可以帮助我们实现对无效参数的有效处理,保证程序的健壮性和稳定性。
相关推荐
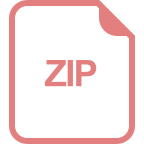
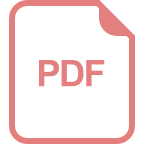
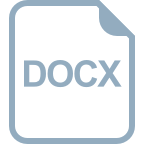












