paddlenlp.datasets.dataset.DatasetBuilder模块如何实现自定义数据集,请代码展示
时间: 2024-04-26 19:27:06 浏览: 12
PaddleNLP 中的 `DatasetBuilder` 模块提供了一个便捷的方式来加载和处理自定义数据集。下面是一个示例代码,展示如何使用 `DatasetBuilder` 自定义数据集:
```python
import os
from paddlenlp.datasets.dataset_builder import DatasetBuilder
# 自定义数据集的路径
data_dir = "/path/to/custom_dataset"
# 定义自己的DatasetBuilder,需要继承DatasetBuilder类
class CustomDataset(DatasetBuilder):
# 数据集的名称,用于指定数据集
# 可以在PaddleNLP中使用的名称有:'chnsenticorp', 'lcqmc', 'msra_ner'
# 如果没有使用PaddleNLP提供的数据集名称,可以使用自己设定的名称
# 在后续的使用中,需要使用DatasetBuilder.get_dataset方法指定数据集名称
NAME = "custom_dataset"
# 数据集的元数据,用于指定数据集的一些基本信息,比如数据集的版本,作者,URL等
META_INFO = {
"version": "1.0.0",
"citation": "Custom Dataset",
"author": "custom_author",
"url": "https://www.custom-dataset.com",
}
# 数据集的文件名称,用于指定数据集的文件名
# 可以是单个文件,也可以是一个文件列表
# 如果是多个文件,需要在read函数中进行合并
SPLITS_FILENAME = {
"train": "train.txt",
"dev": "dev.txt",
"test": "test.txt",
}
# 定义数据集的schema,用于指定数据集的数据格式
# 例如,如果数据集是一个文本分类任务,那么schema可能是{"text": str, "label": int}
# 如果数据集是一个序列标注任务,那么schema可能是{"text": str, "label": List[int]}
# 如果数据集是一个问答任务,那么schema可能是{"context": str, "question": str, "answers": List[str], "label": int}
# 在read函数中,需要将每个样本转换为schema中指定的格式
# 例如,如果数据集是一个文本分类任务,那么每个样本可能是一个字典{"text": "this is a text", "label": 0}
# 在read函数中,需要将每个样本中的"text"和"label"分别提取出来,转换为schema中指定的格式
# 如果不需要提取,可以将schema中的key和value都设为None
# schema = {"text": str, "label": int}
schema = {"text": str, "label": int}
def __init__(self, lazy: bool = True, **kwargs):
"""
自定义数据集的构造函数,需要调用父类的构造函数,并传入一些参数
"""
super().__init__(lazy=lazy, **kwargs)
def _get_data(self, mode, **kwargs):
"""
从数据集文件中读取数据,并返回一个迭代器
"""
# 指定数据集文件路径
file_path = os.path.join(data_dir, self.SPLITS_FILENAME[mode])
# 读取数据,并将每个样本转换为schema中指定的格式
with open(file_path, "r", encoding="utf-8") as f:
for line in f:
text, label = line.strip().split("\t")
yield {"text": text, "label": int(label)}
# 使用自定义数据集
custom_dataset = CustomDataset()
train_ds, dev_ds, test_ds = custom_dataset.get_datasets(["train", "dev", "test"])
```
上述代码中,我们定义了一个自定义数据集 `CustomDataset`,并继承了 `DatasetBuilder` 类。在 `CustomDataset` 中,我们需要指定数据集的名称、元数据、文件名称、数据格式等信息,并在 `_get_data` 函数中读取数据并返回一个迭代器。最后,我们可以使用 `get_datasets` 方法获取数据集的训练集、验证集和测试集。
相关推荐
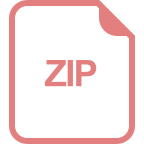
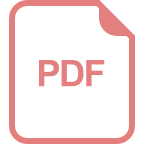
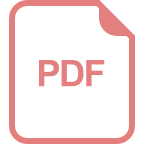














