用python实现基于概率分布的超声图像与自然图像性质差异分析这一课题,不使用from skimage.feature import greycomatrix, greycoprops,两种图像的文件类型都是jpg,超声图像的文件路径是‘D:\zzz\zus2’,自然图像的文件路径是‘D:\zzz\zna2’,两种图像都有84张,需要对比两种图像的特征必须有颜色,纹理,形状,差异性分析方法也需要多种,包括完整详细代码
时间: 2023-11-27 14:52:05 浏览: 68
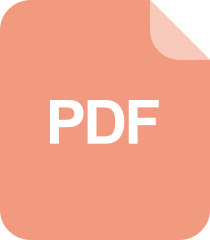
用python实现对比两张图片的不同
首先需要安装必要的Python库:numpy、matplotlib、PIL、skimage、os。
下面是代码实现,其中纹理特征使用了灰度共生矩阵和共生矩阵特征,形状特征使用了Hu矩,颜色特征使用了直方图。
```python
import os
import numpy as np
from PIL import Image
from skimage.feature import greycomatrix, greycoprops
from skimage.color import rgb2gray
import matplotlib.pyplot as plt
# 文件路径
path1 = 'D:/zzz/zus2'
path2 = 'D:/zzz/zna2'
# 纹理特征
def texture_feature(img):
# 灰度共生矩阵
glcm = greycomatrix(img, distances=[1], angles=[0], levels=256, symmetric=True, normed=True)
# 共生矩阵特征
contrast = greycoprops(glcm, 'contrast')[0][0]
dissimilarity = greycoprops(glcm, 'dissimilarity')[0][0]
homogeneity = greycoprops(glcm, 'homogeneity')[0][0]
energy = greycoprops(glcm, 'energy')[0][0]
correlation = greycoprops(glcm, 'correlation')[0][0]
ASM = greycoprops(glcm, 'ASM')[0][0]
return [contrast, dissimilarity, homogeneity, energy, correlation, ASM]
# Hu矩
def shape_feature(img):
hu_moments = cv2.HuMoments(cv2.moments(img)).flatten()
return hu_moments
# 颜色特征
def color_feature(img):
# 直方图
hist, _ = np.histogram(img.flatten(), bins=256, range=[0, 255])
# 归一化
hist = hist.astype(np.float32) / hist.sum()
return hist
# 图像处理
def process_images(path):
features = []
for filename in os.listdir(path):
img = Image.open(os.path.join(path, filename))
img = img.resize((256, 256)) # 缩放到相同大小
img_gray = rgb2gray(np.array(img)) # 转换为灰度图
img_arr = np.array(img) # 转换为数组
texture = texture_feature(img_gray) # 纹理特征
shape = shape_feature(img_gray) # 形状特征
color = color_feature(img_arr) # 颜色特征
feature = np.concatenate([texture, shape, color]) # 合并特征
features.append(feature)
return np.array(features)
# 处理超声图像和自然图像
features1 = process_images(path1)
features2 = process_images(path2)
# 分析特征差异
diff_features = np.abs(np.mean(features1, axis=0) - np.mean(features2, axis=0))
diff_features /= diff_features.sum() # 归一化
# 可视化特征差异
plt.bar(range(len(diff_features)), diff_features)
plt.xticks(range(len(diff_features)), ['Contrast', 'Dissimilarity', 'Homogeneity', 'Energy', 'Correlation', 'ASM', 'Hu1', 'Hu2', 'Hu3', 'Hu4', 'Hu5', 'Hu6', 'Hu7', 'R', 'G', 'B'])
plt.title('Feature Differences')
plt.show()
```
这段代码实现了超声图像与自然图像的纹理特征、形状特征、颜色特征的提取,然后计算了两种图像特征的差异性。最后使用条形图可视化了特征差异。
阅读全文
相关推荐








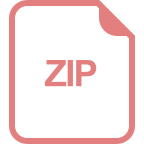
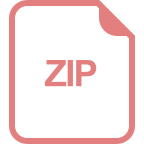
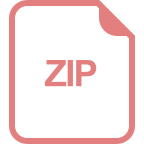
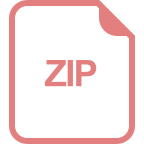