判断给定的字符串是否为回文数组
时间: 2023-12-13 10:31:20 浏览: 150
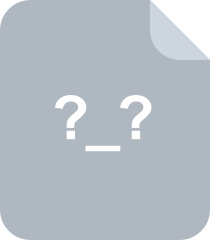
python 回文子串,给你一个字符串a和一个正整数n,判断a中是否存在长度为n的回文子串。

以下是两种判断给定字符串是否为回文的方法:
1.使用循环比较字符串的前后字符是否相同,计数是否占总字符数的一半来判断是否为回文。
```c
#include <stdio.h>
#include <string.h>
int main() {
char str[80];
int i, count = 0;
int t = 0;
gets(str);//输入
count = strlen(str);//统计共有多少个字符
for (i = 0; i < count / 2; i++)//比较总字符数1/2次
{
if (str[i] == str[count - 1 - i]) t++;//若相同,就计数一次
}
if (i == t)//若累计计数达到字符串长度的一半,就是回文
printf("%s是回文\n", str);
else
printf("%s不是回文\n", str);
return 0;
}
```
2.使用栈来判断给定字符串是否为回文。
```javascript
/*使用栈stack类的实现*/
function stack() {
this.dataStore = [];//保存栈内元素,初始化为一个空数组
this.top = 0;//栈顶位置,初始化为0
this.push = push;//入栈
this.pop = pop;//出栈
this.peek = peek;//查看栈顶元素
this.clear = clear;//清空栈
this.length = length;//获取栈内元素个数
}
function push(element) {
this.dataStore[this.top++] = element;
}
function pop() {
return this.dataStore[--this.top];
}
function peek() {
return this.dataStore[this.top - 1];
}
function clear() {
this.top = 0;
}
function length() {
return this.top;
}
function isPalindrome(word) {
var s = new stack();
for (var i = 0; i < word.length; ++i) {
s.push(word[i]);
}
var rword = "";
while (s.length() > 0) {
rword += s.pop();
}
if (word == rword) {
return true;
}
else {
return false;
}
}
var word = "racecar";
if (isPalindrome(word)) {
console.log(word + " is a palindrome.");
}
else {
console.log(word + " is not a palindrome.");
}
```
阅读全文
相关推荐
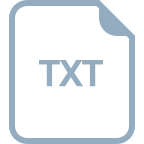
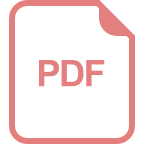

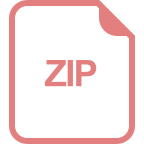

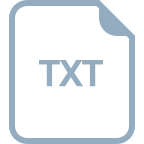
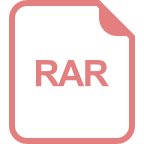
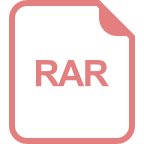
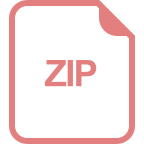






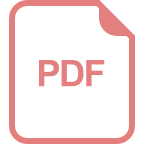
