vue导入element-ui
时间: 2023-10-21 16:28:35 浏览: 187
要导入 Element UI 到 Vue 项目中,你可以按照以下步骤进行操作:
1. 首先,通过 npm 或者 yarn 安装 Element UI 包。在终端中运行以下命令:
```bash
npm install element-ui --save
```
或者
```bash
yarn add element-ui
```
2. 在你的 Vue 项目入口文件(通常是 `main.js`)中,导入 Element UI 的样式和组件。添加以下代码:
```javascript
import Vue from 'vue';
import ElementUI from 'element-ui';
import 'element-ui/lib/theme-chalk/index.css';
Vue.use(ElementUI);
```
3. 然后,你可以在你的 Vue 组件中使用 Element UI 的组件了。例如,在一个.vue 文件中添加一个按钮:
```html
<template>
<div>
<el-button type="primary">这是一个按钮</el-button>
</div>
</template>
```
这样,你就成功地将 Element UI 导入到你的 Vue 项目中了。你可以根据 Element UI 的文档,使用更多的组件和功能。
相关问题
vue安装element-ui
要在Vue项目中安装Element UI库,你可以按照以下步骤操作:
1. **确保已安装Node.js和npm(Node包管理器)**:首先你需要在你的开发环境中安装这两个工具,它们是管理前端依赖的基础。
2. **创建或打开Vue项目**:使用Vue CLI(命令行界面)创建一个新的Vue项目,或者如果你已经有一个项目,导航到项目的根目录。
3. **安装Element UI**:
- 打开终端或命令提示符,切换到你的Vue项目目录。
- 运行`npm install element-ui --save` 或 `yarn add element-ui` 命令。`--save` 或 `-S` 参数会将Element UI添加到项目的`dependencies`或`devDependencies`(取决于你的需求)中。
4. **配置Element UI**:
- 在`main.js`或`src/main.js`文件中,引入Element UI库并注册:
```javascript
import Vue from 'vue';
import ElementUI from 'element-ui';
import 'element-ui/lib/theme-chalk/index.css';
Vue.use(ElementUI);
```
- 请确保已经导入了主题样式,这样Element UI的组件就会根据Chalk主题显示。
5. **开始使用Element UI组件**:
- 现在你可以在Vue组件中使用Element UI提供的各种UI组件,如`<el-button>`, `<el-input>`, 等等。
vue+element-ui实现导入excel的demo
以下是一个基于 Vue 和 Element UI 的导入 Excel 的示例代码:
```vue
<template>
<div>
<el-upload
class="upload-excel"
:before-upload="beforeUpload"
:on-success="onUploadSuccess"
:on-error="onUploadError"
:file-list="fileList"
:accept=".xlsx,.xls"
:auto-upload="false"
>
<el-button slot="trigger" type="primary">选择文件</el-button>
<el-button slot="append" type="success" :disabled="!fileList.length" @click="uploadExcel">上传</el-button>
<div slot="tip" class="el-upload__tip">只能上传 .xlsx 或 .xls 文件</div>
</el-upload>
</div>
</template>
<script>
import XLSX from 'xlsx'
export default {
data() {
return {
fileList: []
}
},
methods: {
beforeUpload(file) {
// 校验文件类型
const isXLS = file.type === 'application/vnd.ms-excel'
const isXLSX = file.type === 'application/vnd.openxmlformats-officedocument.spreadsheetml.sheet'
const isExcel = isXLS || isXLSX
if (!isExcel) {
this.$message.error('只能上传 .xlsx 或 .xls 文件')
return false
}
// 添加到 fileList 中
this.fileList.push(file)
return false // 阻止自动上传
},
onUploadSuccess(response) {
// 上传成功后的处理逻辑
this.$message.success('上传成功!')
},
onUploadError(error) {
// 上传失败后的处理逻辑
this.$message.error('上传失败!')
},
uploadExcel() {
// 读取 Excel 文件内容,此处使用了 xlsx 库
const file = this.fileList[0]
const reader = new FileReader()
reader.onload = (e) => {
const data = e.target.result
const workbook = XLSX.read(data, { type: 'binary' })
const sheetName = workbook.SheetNames[0]
const worksheet = workbook.Sheets[sheetName]
const json = XLSX.utils.sheet_to_json(worksheet)
console.log(json) // 打印 Excel 数据
}
reader.readAsBinaryString(file.raw)
}
}
}
</script>
```
解释一下:
1. 组件中包含一个 `el-upload` 组件,用于上传 Excel 文件。
2. `beforeUpload` 方法用于校验文件类型,并将文件添加到 `fileList` 中。
3. `onUploadSuccess` 和 `onUploadError` 分别是上传成功和上传失败的回调函数。
4. `uploadExcel` 方法用于读取 Excel 文件内容,使用了 `xlsx` 库将 Excel 数据转换成 JSON 数据。
阅读全文
相关推荐
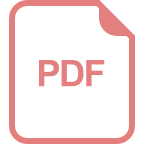
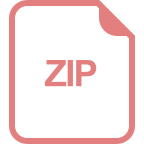
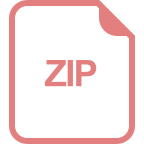
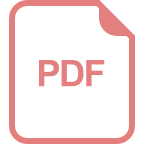
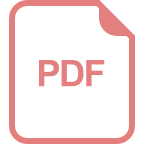
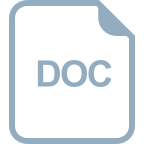
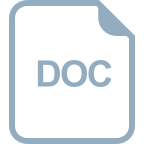
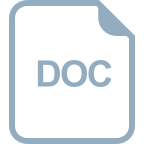





