C#洗牌算法生成不重复的数字
时间: 2023-08-02 17:26:31 浏览: 143
可以使用Fisher-Yates随机置换算法来实现C#洗牌算法生成不重复的数字,具体实现如下:
```csharp
public static int[] Shuffle(int[] arr)
{
Random rand = new Random();
for (int i = arr.Length - 1; i > 0; i--)
{
int j = rand.Next(i + 1);
int temp = arr[i];
arr[i] = arr[j];
arr[j] = temp;
}
return arr;
}
```
这个算法的思路是,从数组的最后一个元素开始,随机选择一个元素与之交换,然后再从剩下的元素中选择一个元素与之交换,以此类推,直到整个数组都被遍历过一遍。这样就可以生成一个随机的、不重复的数组。
相关问题
c# 数组里随机取多个数不重复的
在 C# 中实现从数组中随机取多个数不重复可以使用洗牌算法(Fisher-Yates Shuffle Algorithm)。以下是一个简单的实现:
```csharp
public static T[] Shuffle<T>(T[] array, int count)
{
var random = new Random();
var result = new T[count];
Array.Copy(array, result, count);
for (int i = count; i < array.Length; i++)
{
var j = random.Next(i + 1);
if (j < count)
{
result[j] = array[i];
}
}
return result;
}
```
在上面的代码中,`Shuffle`方法接受两个参数:要随机取数的数组`array`和要取的数的个数`count`。该方法返回一个新的数组,包含从`array`数组中随机取的`count`个不重复的元素。
使用示例:
```csharp
var array = new[] { 1, 2, 3, 4, 5 };
var result = Shuffle(array, 3);
foreach (var item in result)
{
Console.WriteLine(item);
}
```
上面的代码中,`Shuffle`方法将从`array`数组中随机取3个不重复的元素,并将它们存储在`result`数组中。具体的实现方法是,首先将前`count`个元素复制到`result`数组中,然后从`count`到数组末尾进行遍历,对于每个元素,随机生成一个小于等于当前下标的随机数`j`,如果`j`小于`count`,则将该元素存储在`result`数组中的第`j`个位置。这样就可以保证取出的元素不重复,并且随机性较好。
c#中从m个数中随机取出n个不重复的数
在 C# 中,为了从给定的一组 m 个数中随机选取 n 个不重复的数,你可以使用 `System.Linq` 的 `OrderByNew()` 或者 `Zip()` 函数结合 `Random` 类,这里提供一种基于排序的方法:
```csharp
using System;
using System.Collections.Generic;
using System.Linq;
public class Program
{
public static List<int> SelectUnique(int[] numbers, int n)
{
if (numbers.Length < n)
throw new ArgumentException("Not enough numbers to select without repetition.");
// 使用 Fisher-Yates 洗牌算法生成随机排列
Random random = new Random();
for (int i = numbers.Length - 1; i >= n; i--)
{
int j = random.Next(i + 1);
Swap(ref numbers[i], ref numbers[j]);
}
// 取前 n 个元素作为结果
return numbers.Take(n).ToList();
}
private static void Swap(ref int a, ref int b)
{
int temp = a;
a = b;
b = temp;
}
public static void Main(string[] args)
{
int[] numbers = { 1, 2, 3, 4, 5, 6, 7, 8, 9 }; // 例如这里有 m 个数字
int n = 3; // 需要取 n 个不重复的数
var selectedNumbers = SelectUnique(numbers, n);
Console.WriteLine("Selected unique numbers: " + string.Join(", ", selectedNumbers));
}
}
```
这个程序首先检查是否有可能选到不重复的 n 个数,然后对数组进行随机排序,最后取前 n 个元素。运行 `Main` 函数会显示随机选取的不重复的 n 个数。
阅读全文
相关推荐


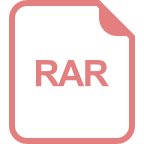
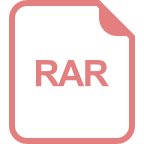







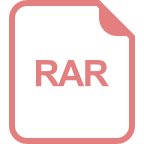
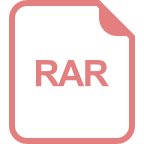

