react native websocket
时间: 2023-05-01 10:03:37 浏览: 496
b'react native websocket' 是一种在 React Native 应用程序中使用的 WebSocket 技术。它允许应用程序与远程服务器之间进行实时通信,可以用于创建实时聊天应用程序、游戏应用程序、实时数据可视化和监控等等。
相关问题
react-native 实现websocket连接并且有心跳检测机制
要实现在 React Native 中建立 WebSocket 连接并进行心跳检测,可以参考以下步骤:
1. 安装 `react-native-websocket` 库
```
npm install --save react-native-websocket
```
2. 在需要使用 WebSocket 的组件中导入并创建 WebSocket 实例,并添加心跳检测逻辑
```javascript
import React, { Component } from 'react';
import { View } from 'react-native';
import WebSocket from 'react-native-websocket';
class MyComponent extends Component {
constructor(props) {
super(props);
this.state = {
ws: null,
};
}
componentDidMount() {
const ws = new WebSocket('ws://localhost:3000');
this.setState({ ws });
// 心跳检测
ws.onopen = () => {
console.log('WebSocket connected');
this.pingInterval = setInterval(() => {
if (ws.readyState === ws.OPEN) {
ws.send(JSON.stringify({ type: 'ping' }));
}
}, 5000);
};
// 接收服务端消息
ws.onmessage = (evt) => {
console.log(`Received message: ${evt.data}`);
};
// WebSocket 断开处理
ws.onclose = () => {
console.log('WebSocket closed');
clearInterval(this.pingInterval);
};
}
componentWillUnmount() {
const { ws } = this.state;
if (ws) {
ws.close();
clearInterval(this.pingInterval);
}
}
render() {
return <View />;
}
}
export default MyComponent;
```
在上述代码中,我们创建了一个 WebSocket 实例,并在 `componentDidMount` 生命周期钩子中添加了心跳检测逻辑。这里我们使用了 `setInterval` 定时发送 `ping` 消息,并在 `onmessage` 回调中处理服务端返回的消息。在 `onclose` 回调中清除定时器。
3. 注意事项
- 在组件卸载时,需要关闭 WebSocket 连接并清除心跳检测定时器,以免引起内存泄漏;
- 心跳检测的时间间隔需要根据具体业务需求进行调整;
- 需要注意处理 WebSocket 连接异常情况,例如网络异常或服务端关闭连接等;
react-native 使用websocket并带有心跳检测机制与重新连接机制
在 React Native 中使用 WebSocket,可以使用内置的 `WebSocket` API。要实现心跳检测和重新连接机制,可以编写自定义的 WebSocket 类。
下面是一个简单的实现,可以用作参考:
```javascript
class MyWebSocket {
constructor(url) {
this.ws = new WebSocket(url);
this.heartBeatTimer = null;
this.reconnectTimer = null;
this.isClosed = false;
this.ws.onopen = () => {
this.heartBeat();
};
this.ws.onmessage = (event) => {
// 处理收到的消息
};
this.ws.onerror = (error) => {
console.log(`WebSocket error: ${error}`);
this.reconnect();
};
this.ws.onclose = () => {
console.log(`WebSocket closed`);
this.isClosed = true;
this.reconnect();
};
}
send(data) {
if (!this.isClosed) {
this.ws.send(data);
}
}
heartBeat() {
this.send('heart beat');
this.heartBeatTimer = setTimeout(() => {
this.heartBeat();
}, 10000); // 10秒检测一次心跳
}
reconnect() {
if (this.isClosed) {
return;
}
if (this.reconnectTimer) {
clearTimeout(this.reconnectTimer);
}
this.reconnectTimer = setTimeout(() => {
console.log(`WebSocket reconnecting...`);
this.ws = new WebSocket(this.ws.url);
this.ws.onopen = () => {
console.log(`WebSocket reconnected`);
clearTimeout(this.reconnectTimer);
this.isClosed = false;
this.heartBeat();
};
this.ws.onmessage = (event) => {
// 处理收到的消息
};
this.ws.onerror = (error) => {
console.log(`WebSocket error: ${error}`);
this.reconnect();
};
this.ws.onclose = () => {
console.log(`WebSocket closed`);
this.isClosed = true;
this.reconnect();
};
}, 3000); // 3秒后尝试重新连接
}
close() {
this.isClosed = true;
clearTimeout(this.heartBeatTimer);
clearTimeout(this.reconnectTimer);
this.ws.close();
}
}
```
这个自定义的 WebSocket 类具有心跳检测和重新连接机制。在初始化时,会创建一个 WebSocket 连接,并设置 `onopen`、`onmessage`、`onerror` 和 `onclose` 事件的回调函数。当连接打开时,会开始心跳检测;当连接关闭时,会尝试重新连接。在发送消息时,会检查连接是否已关闭,如果未关闭,则发送消息。关闭连接时,会清除定时器并关闭 WebSocket 连接。
使用这个自定义的 WebSocket 类时,只需实例化它,并调用其 `send` 方法发送消息:
```javascript
const ws = new MyWebSocket('ws://localhost:8080');
ws.send('hello');
```
也可以在需要时关闭连接:
```javascript
ws.close();
```
这样就可以在 React Native 中使用 WebSocket,并实现心跳检测和重新连接机制了。
阅读全文
相关推荐
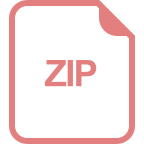
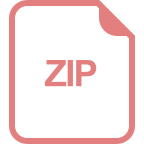
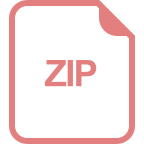
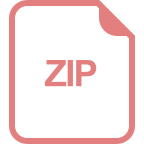
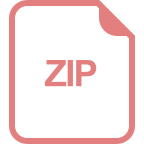
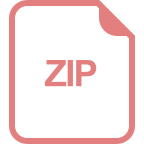
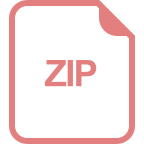
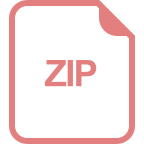
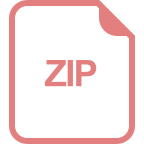
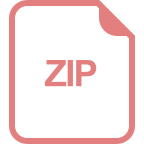
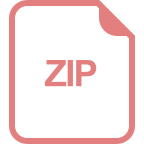
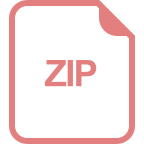
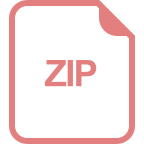
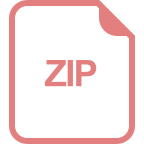
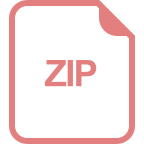
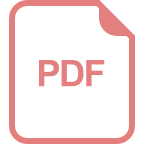
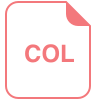