vue中table表格显示全选
时间: 2023-06-14 22:05:45 浏览: 145
在 Vue 中实现 table 表格全选功能,可以通过以下步骤:
1. 在 data 中定义一个变量,用来表示是否全选,例如 `selectAll: false`
2. 在 table 表格中添加一个全选的 checkbox,绑定到 selectAll 变量上,例如:
```
<thead>
<tr>
<th><input type="checkbox" v-model="selectAll"></th>
<th>Column 1</th>
<th>Column 2</th>
<th>Column 3</th>
</tr>
</thead>
```
3. 在每一行的 checkbox 中绑定一个 selected 属性,用来表示该行是否被选中,例如:
```
<tbody>
<tr v-for="(item, index) in list" :key="index">
<td><input type="checkbox" v-model="item.selected"></td>
<td>{{ item.column1 }}</td>
<td>{{ item.column2 }}</td>
<td>{{ item.column3 }}</td>
</tr>
</tbody>
```
4. 监听 selectAll 变量的变化,在其变为 true 时将所有行的 selected 属性都设置为 true,在其变为 false 时将所有行的 selected 属性都设置为 false,例如:
```
watch: {
selectAll: function(val) {
this.list.forEach(function(item) {
item.selected = val;
});
}
}
```
5. 实现全选和取消全选的方法,例如:
```
methods: {
selectAllRows: function() {
this.selectAll = true;
},
deselectAllRows: function() {
this.selectAll = false;
}
}
```
将这两个方法绑定到全选和取消全选的按钮上即可。
完整代码示例:
```
<template>
<div>
<button @click="selectAllRows">Select All</button>
<button @click="deselectAllRows">Deselect All</button>
<table>
<thead>
<tr>
<th><input type="checkbox" v-model="selectAll"></th>
<th>Column 1</th>
<th>Column 2</th>
<th>Column 3</th>
</tr>
</thead>
<tbody>
<tr v-for="(item, index) in list" :key="index">
<td><input type="checkbox" v-model="item.selected"></td>
<td>{{ item.column1 }}</td>
<td>{{ item.column2 }}</td>
<td>{{ item.column3 }}</td>
</tr>
</tbody>
</table>
</div>
</template>
<script>
export default {
data() {
return {
selectAll: false,
list: [
{ column1: 'Row 1 Column 1', column2: 'Row 1 Column 2', column3: 'Row 1 Column 3', selected: false },
{ column1: 'Row 2 Column 1', column2: 'Row 2 Column 2', column3: 'Row 2 Column 3', selected: false },
{ column1: 'Row 3 Column 1', column2: 'Row 3 Column 2', column3: 'Row 3 Column 3', selected: false }
]
}
},
watch: {
selectAll: function(val) {
this.list.forEach(function(item) {
item.selected = val;
});
}
},
methods: {
selectAllRows: function() {
this.selectAll = true;
},
deselectAllRows: function() {
this.selectAll = false;
}
}
}
</script>
```
阅读全文
相关推荐
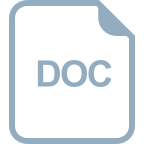
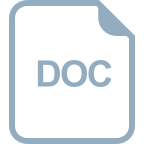
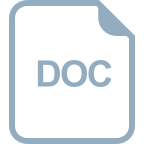




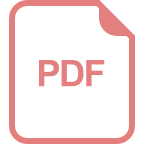










