wpf mvvm实现 listbox上移下移
时间: 2023-08-01 20:14:23 浏览: 143
在 WPF MVVM 中,可以通过使用 `ICommand` 和 `RelayCommand` 来实现 `ListBox` 中的上移和下移操作。
以下是一个实现上移和下移功能的示例代码:
1. 在视图模型中,创建一个 `ObservableCollection` 来存储列表项,并创建两个 `RelayCommand` 分别用于上移和下移操作:
```
public class MyViewModel : INotifyPropertyChanged
{
private ObservableCollection<string> _items = new ObservableCollection<string>();
public ObservableCollection<string> Items
{
get { return _items; }
set
{
_items = value;
OnPropertyChanged(nameof(Items));
}
}
public ICommand MoveUpCommand { get; set; }
public ICommand MoveDownCommand { get; set; }
public MyViewModel()
{
MoveUpCommand = new RelayCommand<object>(MoveUp, CanMoveUp);
MoveDownCommand = new RelayCommand<object>(MoveDown, CanMoveDown);
}
private bool CanMoveUp(object parameter)
{
var index = Items.IndexOf(parameter as string);
return index > 0;
}
private void MoveUp(object parameter)
{
var index = Items.IndexOf(parameter as string);
var temp = Items[index];
Items[index] = Items[index - 1];
Items[index - 1] = temp;
}
private bool CanMoveDown(object parameter)
{
var index = Items.IndexOf(parameter as string);
return index >= 0 && index < Items.Count - 1;
}
private void MoveDown(object parameter)
{
var index = Items.IndexOf(parameter as string);
var temp = Items[index];
Items[index] = Items[index + 1];
Items[index + 1] = temp;
}
public event PropertyChangedEventHandler PropertyChanged;
protected void OnPropertyChanged(string propertyName)
{
PropertyChanged?.Invoke(this, new PropertyChangedEventArgs(propertyName));
}
}
```
2. 在视图中,使用 `ListBox` 来显示列表项,并绑定上移和下移命令:
```
<ListBox ItemsSource="{Binding Items}">
<ListBox.ItemTemplate>
<DataTemplate>
<StackPanel Orientation="Horizontal">
<Button Content="▲" Command="{Binding DataContext.MoveUpCommand, RelativeSource={RelativeSource AncestorType={x:Type ListBox}}}" CommandParameter="{Binding}" />
<Button Content="▼" Command="{Binding DataContext.MoveDownCommand, RelativeSource={RelativeSource AncestorType={x:Type ListBox}}}" CommandParameter="{Binding}" />
<TextBlock Text="{Binding}" />
</StackPanel>
</DataTemplate>
</ListBox.ItemTemplate>
</ListBox>
```
在上面的示例中,使用了 `RelayCommand` 类来实现命令的绑定。它是一个实现了 `ICommand` 接口的类,可以用于在视图模型中声明和实现命令。在 `ListBox` 的数据模板中,使用了 `RelativeSource` 来绑定 `ListBox` 的父级元素(即视图),以便使用视图模型中的命令。
阅读全文
相关推荐
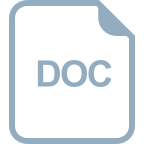
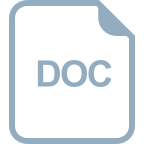
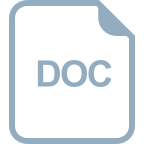

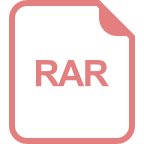
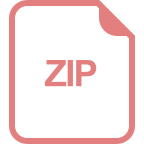
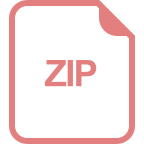
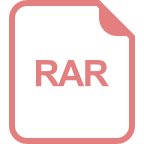
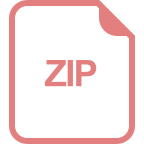
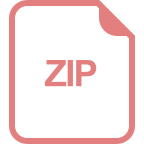
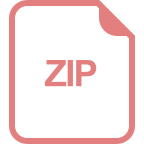
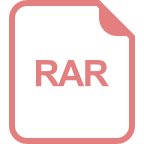
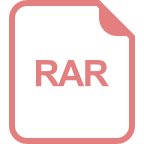
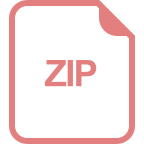
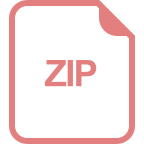
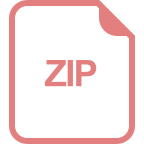