python日计复利
时间: 2023-11-15 18:01:50 浏览: 47
Python可以用来计算复利。复利是指在一定时间内,本金和利息再次计算利息的过程。Python程序可以根据给定的原理量、速率和时间来计算复利。下面是一个Python程序示例,可以计算复利:
```python
# Python程序找到复利 (Python program to find compound interest)
p = float(input("Enter the principle amount : "))
r = float(input("Enter the rate of interest : "))
t = float(input("Enter the time in the years: "))
# 计算复利
ci = p * (pow((1 + r / 100), t))
# 打印结果
print("Principle amount : ", p)
print("Interest rate : ", r)
print("Time in years : ", t)
print("Compound Interest : ", ci)
```
在这个程序中,用户需要输入原理量、速率和时间。程序会使用这些值来计算复利,并将结果打印出来。
相关问题
用python表示连续复利
可以使用以下代码来表示连续复利:
```python
def continuous_compounding(principal, rate, time):
return principal * (1 + rate) ** time
```
其中,principal表示本金,rate表示年利率,time表示投资时间(单位为年)。
python 复利 年金
复利年金是指在每一期的期末支付等额的一笔现金,并将该现金继续投资,以便在未来获得更多的回报。在Python中,我们可以使用以下数学公式来计算复利年金的现值:
def pv_f(fv, r, n):
pv = fv / ((1 + r) ** n)
return pv
其中,fv表示未来年金的金额,r表示每一期的利率,n表示总的期数。
例如,假设未来年金金额为100,年利率为0.1,总的期数为2,我们可以使用上述函数来计算复利年金的现值:
pv = pv_f(100, 0.1, 2)
结果为82.64 [1。
请注意,复利年金的计算需要考虑每一期的利息累积效应,因此在计算现值时需要将未来年金金额除以每一期的利率的幂次。
希望以上回答能够解决你的问题。如果还有其他问题,请随时提问。<span class="em">1</span><span class="em">2</span><span class="em">3</span>
#### 引用[.reference_title]
- *1* *2* *3* [Pycharm 金融Python实战二:用Python编写一个金融计算器——编写函数 调用命令 实例年金现值 利率换算 净...](https://blog.csdn.net/tianshijie08/article/details/120423804)[target="_blank" data-report-click={"spm":"1018.2226.3001.9630","extra":{"utm_source":"vip_chatgpt_common_search_pc_result","utm_medium":"distribute.pc_search_result.none-task-cask-2~all~insert_cask~default-1-null.142^v92^chatsearchT3_1"}}] [.reference_item style="max-width: 100%"]
[ .reference_list ]
相关推荐
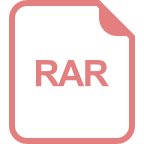












