银行家算法java代码实现
时间: 2023-08-14 18:35:24 浏览: 55
以下是银行家算法的Java代码实现,其中包括了安全性检查和资源分配过程:
```java
import java.util.*;
public class BankerAlgorithm {
private int[][] maximum; // 最大需求矩阵
private int[][] allocation; // 已分配矩阵
private int[][] need; // 需求矩阵
private int[] available; // 可用资源向量
private int[] work; // 工作向量
private boolean[] finish; // 进程完成标志
public BankerAlgorithm(int[][] max, int[][] alloc, int[] avail) {
int n = max.length; // 进程数
int m = avail.length; // 资源种类数
maximum = new int[n][m];
allocation = new int[n][m];
need = new int[n][m];
available = new int[m];
work = new int[m];
finish = new boolean[n];
for (int i = 0; i < n; i++) {
for (int j = 0; j < m; j++) {
maximum[i][j] = max[i][j];
allocation[i][j] = alloc[i][j];
need[i][j] = max[i][j] - alloc[i][j];
}
}
for (int j = 0; j < m; j++) {
available[j] = avail[j];
work[j] = avail[j];
}
}
// 检查当前状态是否安全
public boolean isSafeState() {
int n = finish.length; // 进程数
int m = available.length; // 资源种类数
int count = 0; // 已完成进程数
boolean[] safe = new boolean[n]; // 安全序列
Arrays.fill(safe, false);
while (count < n) {
boolean flag = false;
for (int i = 0; i < n; i++) {
if (!finish[i]) {
int j;
for (j = 0; j < m; j++) {
if (need[i][j] > work[j]) {
break;
}
}
if (j == m) {
for (j = 0; j < m; j++) {
work[j] += allocation[i][j];
}
safe[count++] = true;
finish[i] = true;
flag = true;
}
}
}
if (!flag) {
break;
}
}
if (count < n) {
return false; // 不安全状态
} else {
System.out.print("安全序列:");
for (int i = 0; i < n; i++) {
System.out.print("P" + (safe[i] ? i : -1) + " ");
}
System.out.println();
return true; // 安全状态
}
}
// 分配资源
public boolean requestResources(int pid, int[] req) {
int m = available.length; // 资源种类数
if (pid < 0 || pid >= maximum.length) {
return false; // 进程不存在
}
for (int j = 0; j < m; j++) {
if (req[j] > need[pid][j] || req[j] > available[j]) {
return false; // 请求资源超出需求或可用量
}
}
for (int j = 0; j < m; j++) {
available[j] -= req[j];
allocation[pid][j] += req[j];
need[pid][j] -= req[j];
work[j] = available[j];
}
boolean safe = isSafeState(); // 检查安全性
if (!safe) { // 恢复原来状态
for (int j = 0; j < m; j++) {
available[j] += req[j];
allocation[pid][j] -= req[j];
need[pid][j] += req[j];
work[j] = available[j];
}
}
return safe;
}
// 释放资源
public void releaseResources(int pid) {
int m = available.length; // 资源种类数
if (pid < 0 || pid >= maximum.length) {
return; // 进程不存在
}
for (int j = 0; j < m; j++) {
available[j] += allocation[pid][j];
allocation[pid][j] = 0;
need[pid][j] = maximum[pid][j];
work[j] = available[j];
}
}
// 显示状态矩阵
public void showStatus() {
System.out.println("最大需求矩阵:");
showMatrix(maximum);
System.out.println("已分配矩阵:");
showMatrix(allocation);
System.out.println("需求矩阵:");
showMatrix(need);
System.out.println("可用资源向量:");
showVector(available);
System.out.println("工作向量:");
showVector(work);
System.out.println("进程完成标志:");
showVector(finish);
}
// 显示矩阵
private void showMatrix(int[][] matrix) {
for (int[] row : matrix) {
for (int cell : row) {
System.out.print(cell + " ");
}
System.out.println();
}
}
// 显示向量
private void showVector(int[] vector) {
for (int cell : vector) {
System.out.print(cell + " ");
}
System.out.println();
}
public static void main(String[] args) {
int[][] max = { { 7, 5, 3 }, { 3, 2, 2 }, { 9, 0, 2 }, { 2, 2, 2 }, { 4, 3, 3 } };
int[][] alloc = { { 0, 1, 0 }, { 2, 0, 0 }, { 3, 0, 2 }, { 2, 1, 1 }, { 0, 0, 2 } };
int[] avail = { 3, 3, 2 };
BankerAlgorithm bank = new BankerAlgorithm(max, alloc, avail);
bank.showStatus();
System.out.println();
bank.isSafeState();
System.out.println();
bank.requestResources(1, new int[] { 1, 0, 2 });
bank.showStatus();
System.out.println();
bank.isSafeState();
System.out.println();
bank.requestResources(4, new int[] { 0, 2, 0 });
bank.showStatus();
System.out.println();
bank.isSafeState();
}
}
```
示例代码中,我们以一个包含 5 个进程和 3 种资源的系统为例进行演示。在主函数中,我们先构造一个银行家算法的实例,然后调用它的方法完成安全性检查和资源分配过程。
相关推荐
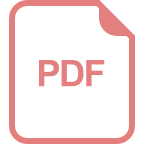
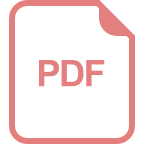














