用java写餐饮管理系统
时间: 2023-11-14 21:07:00 浏览: 63
餐饮管理系统是一个复杂的系统,需要涉及到多个模块,包括菜单管理、订单管理、库存管理、员工管理等。以下是一个简单的餐饮管理系统的Java代码示例。
首先,我们需要定义一些基本的实体类:
```java
public class Food {
private int id;
private String name;
private double price;
public Food(int id, String name, double price) {
this.id = id;
this.name = name;
this.price = price;
}
// getters and setters
}
public class Order {
private int id;
private List<Food> foods;
private double total;
public Order(int id, List<Food> foods, double total) {
this.id = id;
this.foods = foods;
this.total = total;
}
// getters and setters
}
public class Employee {
private int id;
private String name;
private String position;
public Employee(int id, String name, String position) {
this.id = id;
this.name = name;
this.position = position;
}
// getters and setters
}
public class Inventory {
private int id;
private Food food;
private int quantity;
public Inventory(int id, Food food, int quantity) {
this.id = id;
this.food = food;
this.quantity = quantity;
}
// getters and setters
}
```
然后,我们需要实现各个模块的功能,例如:
```java
public class MenuManager {
private List<Food> foods;
public MenuManager() {
this.foods = new ArrayList<>();
}
public void addFood(Food food) {
this.foods.add(food);
}
public void removeFood(Food food) {
this.foods.remove(food);
}
public List<Food> getFoods() {
return this.foods;
}
}
public class OrderManager {
private List<Order> orders;
public OrderManager() {
this.orders = new ArrayList<>();
}
public void addOrder(Order order) {
this.orders.add(order);
}
public void removeOrder(Order order) {
this.orders.remove(order);
}
public List<Order> getOrders() {
return this.orders;
}
}
public class InventoryManager {
private List<Inventory> inventories;
public InventoryManager() {
this.inventories = new ArrayList<>();
}
public void addInventory(Inventory inventory) {
this.inventories.add(inventory);
}
public void removeInventory(Inventory inventory) {
this.inventories.remove(inventory);
}
public List<Inventory> getInventories() {
return this.inventories;
}
public int getInventoryQuantity(Food food) {
for (Inventory inventory : this.inventories) {
if (inventory.getFood().equals(food)) {
return inventory.getQuantity();
}
}
return 0;
}
public void updateInventoryQuantity(Food food, int quantity) {
for (Inventory inventory : this.inventories) {
if (inventory.getFood().equals(food)) {
inventory.setQuantity(quantity);
}
}
}
}
public class EmployeeManager {
private List<Employee> employees;
public EmployeeManager() {
this.employees = new ArrayList<>();
}
public void addEmployee(Employee employee) {
this.employees.add(employee);
}
public void removeEmployee(Employee employee) {
this.employees.remove(employee);
}
public List<Employee> getEmployees() {
return this.employees;
}
}
```
最后,我们可以编写一个简单的主程序来模拟餐饮管理系统的使用:
```java
public class RestaurantMain {
public static void main(String[] args) {
// 初始化菜单
MenuManager menuManager = new MenuManager();
menuManager.addFood(new Food(1, "烤鸭", 88.0));
menuManager.addFood(new Food(2, "糖醋排骨", 38.0));
menuManager.addFood(new Food(3, "宫保鸡丁", 28.0));
// 初始化库存
InventoryManager inventoryManager = new InventoryManager();
inventoryManager.addInventory(new Inventory(1, menuManager.getFoods().get(0), 10));
inventoryManager.addInventory(new Inventory(2, menuManager.getFoods().get(1), 20));
inventoryManager.addInventory(new Inventory(3, menuManager.getFoods().get(2), 30));
// 初始化员工
EmployeeManager employeeManager = new EmployeeManager();
employeeManager.addEmployee(new Employee(1, "张三", "服务员"));
employeeManager.addEmployee(new Employee(2, "李四", "厨师"));
// 模拟点餐流程
List<Food> foods = new ArrayList<>();
foods.add(menuManager.getFoods().get(0));
foods.add(menuManager.getFoods().get(1));
Order order = new Order(1, foods, 126.0);
inventoryManager.updateInventoryQuantity(menuManager.getFoods().get(0), inventoryManager.getInventoryQuantity(menuManager.getFoods().get(0)) - 1);
inventoryManager.updateInventoryQuantity(menuManager.getFoods().get(1), inventoryManager.getInventoryQuantity(menuManager.getFoods().get(1)) - 1);
employeeManager.getEmployees().get(0).takeOrder(order);
employeeManager.getEmployees().get(1).prepareFood(order);
// 模拟结账流程
employeeManager.getEmployees().get(0).checkout(order);
}
}
```
当然,这只是一个简单的示例,实际的餐饮管理系统要比这个复杂得多。但是,通过这个示例,您可以了解到如何使用Java编写一个基本的餐饮管理系统。
相关推荐
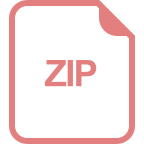
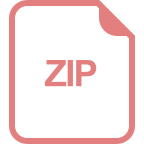
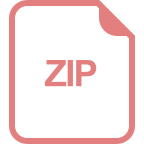














