写出一个互斥双buff读写接口
时间: 2023-12-02 22:06:26 浏览: 43
以下是一个简单的互斥双缓冲读写接口的示例代码,其中使用了C++11中的互斥锁std::mutex和条件变量std::condition_variable来实现线程同步。
```c++
#include <iostream>
#include <mutex>
#include <condition_variable>
#include <thread>
#include <chrono>
// 互斥双缓冲区结构体
template<typename T>
struct double_buffer {
std::mutex mtx; // 互斥锁
std::condition_variable cv; // 条件变量
T* read_buffer; // 读缓冲区指针
T* write_buffer; // 写缓冲区指针
size_t size; // 缓冲区大小
bool data_ready; // 数据是否准备好
bool stop; // 线程是否停止
// 构造函数
double_buffer(size_t size) : size(size), data_ready(false), stop(false) {
read_buffer = new T[size];
write_buffer = new T[size];
}
// 析构函数
~double_buffer() {
delete[] read_buffer;
delete[] write_buffer;
}
// 读取数据
void read(T* buffer, size_t count) {
std::unique_lock<std::mutex> lock(mtx); // 上锁
while (!data_ready && !stop) { // 等待数据准备好或线程停止
cv.wait(lock);
}
if (data_ready) { // 从读缓冲区复制数据
memcpy(buffer, read_buffer, count * sizeof(T));
data_ready = false;
cv.notify_all();
}
}
// 写入数据
void write(T* buffer, size_t count) {
std::unique_lock<std::mutex> lock(mtx); // 上锁
while (data_ready && !stop) { // 等待数据被读取或线程停止
cv.wait(lock);
}
if (!stop) { // 写入数据到写缓冲区
memcpy(write_buffer, buffer, count * sizeof(T));
data_ready = true;
std::swap(read_buffer, write_buffer); // 交换读写缓冲区指针
cv.notify_all();
}
}
// 停止线程
void stop_thread() {
stop = true;
cv.notify_all();
}
};
// 测试函数
void test_double_buffer() {
double_buffer<int> db(10); // 创建双缓冲区
std::thread t([&]() { // 创建线程写数据
int data[10];
for (int i = 0; i < 100; ++i) {
for (int j = 0; j < 10; ++j) {
data[j] = i * 10 + j;
}
db.write(data, 10);
std::this_thread::sleep_for(std::chrono::milliseconds(10));
}
db.stop_thread();
});
int buffer[10];
while (true) { // 循环读数据
db.read(buffer, 10);
for (int i = 0; i < 10; ++i) {
std::cout << buffer[i] << " ";
}
std::cout << std::endl;
if (db.stop) {
break;
}
}
t.join(); // 等待线程结束
}
// 主函数
int main() {
test_double_buffer();
return 0;
}
```
该示例代码中,使用double_buffer结构体来实现互斥双缓冲区的读写功能。其中,互斥锁和条件变量用来保证线程同步,read_buffer和write_buffer分别用来保存读缓冲区和写缓冲区的数据,data_ready表示数据是否准备好,stop表示线程是否停止。在read函数中,线程会等待数据准备好或线程停止,然后从读缓冲区复制数据到用户缓冲区,并将data_ready标记为false,最后唤醒其他等待线程。在write函数中,线程会等待数据被读取或线程停止,然后将用户缓冲区的数据复制到写缓冲区,并将data_ready标记为true,然后交换读写缓冲区指针,并唤醒其他等待线程。在stop_thread函数中,线程会将stop标记为true,并唤醒其他等待线程,以停止线程的运行。在主函数中,通过调用test_double_buffer函数来测试双缓冲区的读写功能。
相关推荐
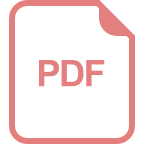














