写出使用STM32STM32F103RCT6的SPI1读写SD卡的全部函数
时间: 2024-04-12 11:32:16 浏览: 257
使用STM32F103RCT6通过SPI1读写SD卡的函数如下所示:
1. 初始化SD卡:
```c
void SD_Init(void) {
// 初始化SPI1
SPI1_Init();
// 初始化SD卡
SD_SendCommand(CMD0, 0x00, 0x95); // 发送复位命令
// 等待SD卡初始化完成
while (SD_SendCommand(CMD1, 0x00, 0xFF) != 0x00);
}
```
2. 发送一个字节到SD卡并接收响应:
```c
uint8_t SD_SendByte(uint8_t byte) {
while (SPI_I2S_GetFlagStatus(SPI1, SPI_I2S_FLAG_TXE) == RESET);
SPI_I2S_SendData(SPI1, byte);
while (SPI_I2S_GetFlagStatus(SPI1, SPI_I2S_FLAG_RXNE) == RESET);
return SPI_I2S_ReceiveData(SPI1);
}
```
3. 发送SD卡命令:
```c
uint8_t SD_SendCommand(uint8_t cmd, uint32_t arg, uint8_t crc) {
uint8_t response;
// 发送命令字节
SD_CS_LOW();
SD_SendByte(cmd | 0x40);
// 发送参数
SD_SendByte(arg >> 24);
SD_SendByte(arg >> 16);
SD_SendByte(arg >> 8);
SD_SendByte(arg);
// 发送CRC校验字节
SD_SendByte(crc);
// 接收响应字节
while ((response = SD_SendByte(0xFF)) == 0xFF);
// 收到响应
return response;
}
```
4. 读取SD卡数据块:
```c
uint8_t SD_ReadBlock(uint32_t sector, uint8_t* buffer) {
uint16_t i;
uint8_t response;
uint8_t dataToken;
// 发送读命令
response = SD_SendCommand(CMD17, sector << 9, 0xFF);
// 响应错误
if (response != 0x00) {
return response;
}
// 找到数据起始令牌
while (SD_SendByte(0xFF) != 0xFE);
// 读取数据
for (i = 0; i < 512; i++) {
buffer[i] = SD_SendByte(0xFF);
}
// 读取2个CRC校验字节
SD_SendByte(0xFF);
SD_SendByte(0xFF);
// 读取完毕,释放SD卡
SD_CS_HIGH();
return 0;
}
```
5. 写入SD卡数据块:
```c
uint8_t SD_WriteBlock(uint32_t sector, const uint8_t* buffer) {
uint16_t i;
uint8_t response;
// 发送写命令
response = SD_SendCommand(CMD24, sector << 9, 0xFF);
// 响应错误
if (response != 0x00) {
return response;
}
// 发送数据起始令牌
SD_CS_LOW();
SD_SendByte(0xFE);
// 发送数据
for (i = 0; i < 512; i++) {
SD_SendByte(buffer[i]);
}
// 发送2个CRC校验字节
SD_SendByte(0xFF);
SD_SendByte(0xFF);
// 接收响应
response = SD_SendByte(0xFF);
// 响应为数据写入完成
if ((response & 0x1F) != 0x05) {
// 写入失败,释放SD卡
SD_CS_HIGH();
return response;
}
// 等待SD卡写入完成
while (SD_SendByte(0xFF) != 0xFF);
// 写入成功,释放SD卡
SD_CS_HIGH();
return 0;
}
```
以上函数提供了基本的SD卡读写功能,可以根据具体需求进行进一步扩展和优化。
阅读全文
相关推荐


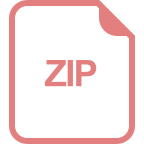
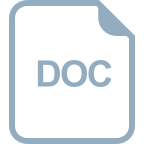
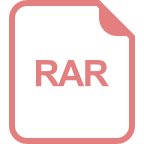
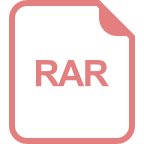
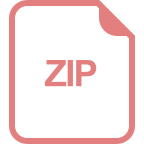
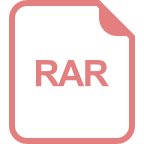
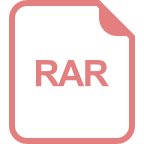
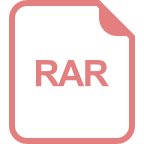
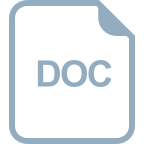
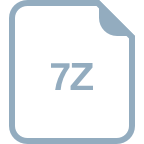
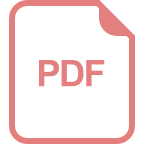