django 实时显示adb logcat日志
时间: 2023-05-26 11:02:31 浏览: 169
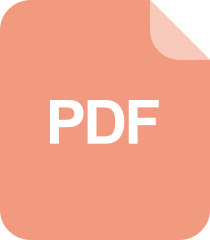
Django实现的自定义访问日志模块示例
要在 Django 中实时显示 adb logcat 日志,可以通过以下步骤进行操作:
1. 在 Django 项目的 settings.py 文件中添加以下代码,以启用子进程功能:
```python
import subprocess
from threading import Thread
from time import sleep
def run_logcat():
cmd = ['adb', 'logcat']
p = subprocess.Popen(cmd, stdout=subprocess.PIPE, stderr=subprocess.PIPE)
for line in iter(p.stdout.readline, b''):
yield line.decode()
def logcat_generator():
for line in run_logcat():
yield line
class LogcatThread(Thread):
daemon = True
def run(self):
with open('logcat.log', 'w') as f:
for line in logcat_generator():
f.write(line)
```
2. 在 URLconf 中添加以下代码,以启动线程并按需访问生成的日志文件:
```python
from django.urls import path
from django.http import StreamingHttpResponse
from . import views
urlpatterns = [
path('', views.index),
path('logcat/', views.logcat),
]
def logcat_response():
def generate():
with open('logcat.log') as f:
for line in f:
yield line.encode()
return StreamingHttpResponse(generate())
LogcatThread().start()
```
3. 在 views.py 文件中添加以下视图处理程序:
```python
from django.shortcuts import render
def index(request):
return render(request, 'index.html')
def logcat(request):
return logcat_response()
```
4. 创建一个名为 index.html 的模板,其中包含一个触发器来请求日志:
```html
{% extends 'base.html' %}
{% block content %}
<h1>ADB Logcat Viewer</h1>
<p>You can view the logcat messages by clicking the following button:</p>
<button onclick="reloadLogcat()">Refresh Logcat</button>
<pre id="logcat"></pre>
{% endblock %}
{% block javascript %}
<script type="text/javascript">
function reloadLogcat() {
var xhr = new XMLHttpRequest();
xhr.onreadystatechange = function () {
if (this.readyState == 4 && this.status == 200) {
document.getElementById("logcat").innerText = this.responseText;
}
};
xhr.open("GET", "/logcat/");
xhr.send();
}
</script>
{% endblock %}
```
现在, 每次您访问网站并单击“刷新 Logcat”按钮时,您将获得最新的 adb logcat 日志。
阅读全文
相关推荐
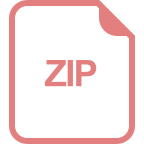
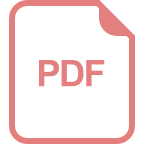














