C语言程序设计:计算某年某月某日是该年的第几天
时间: 2024-09-16 08:03:16 浏览: 60
在C语言中,计算给定日期是一年中的第几天,通常需要考虑闰年的情况。下面是一个简单的步骤:
1. 确定月份和日子:假设输入的是`day`, `month`, 和 `year`变量。
2. 对于一月和二月,特殊处理:
- 如果是1月,且该年是闰年(能被4整除,但是不能被100整除,除非同时能被400整除),那么第一天是32天;
- 否则,如果1月1日是该年的第一天,那么它是31天;
- 否则,即上一年的最后一天是12月31日,则是31天。
3. 对于其他月份(从3月到12月),每个月的天数固定:
- 3月到11月,每月都是31天;
- 12月如果是非闰年,有31天;如果是闰年,有30天。
4. 使用循环计算总天数,从1月开始累加直到目标月,加上目标月的日数。
5. 最后,检查是否是闰年,调整二月的天数。闰年的判断规则:年份能被4整除且不能被100整除,或者可以被400整除。
这是一个基础版本的算法,实际编程时可能需要编写函数并包含对输入错误的验证。下面是伪代码示例:
```c
bool is_leap_year(int year) {
return (year % 4 == 0 && year % 100 != 0) || (year % 400 == 0);
}
int days_in_month(int month, int year) {
if (month == 2) {
return is_leap_year(year) ? 29 : 28;
} else if (month > 2) {
return 31; // 除了2月,其他都是31天
} else {
return 31; // January has 31 days
}
}
int day_of_year(int day, int month, int year) {
int total_days = 0;
for (int i = 1; i < month; ++i) {
total_days += days_in_month(i, year);
}
total_days += day;
return total_days;
}
```
阅读全文
相关推荐
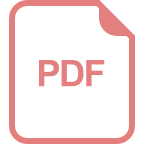
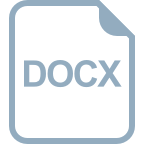
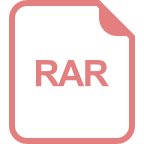















