C语言编写程序 输入某年某月某日,判断这一天是这一年的第几天
时间: 2024-10-24 11:14:48 浏览: 102
在C语言中,编写一个函数来计算给定日期是一年中的第几天,需要考虑闰年的情况,因为二月有28天或29天。以下是一个简单的示例,这个函数名为`days_in_month()`,它接收年份、月份和日期作为输入:
```c
#include <stdio.h>
// 检查是否是闰年
int is_leap_year(int year) {
return (year % 4 == 0 && year % 100 != 0) || (year % 400 == 0);
}
// 计算一个月中的天数
int days_in_month(int month, int year) {
switch (month) {
case 1: case 3: case 5: case 7: case 8: case 10: case 12:
return 31;
case 4: case 6: case 9: case 11:
return 30;
case 2:
if (is_leap_year(year)) {
return 29;
} else {
return 28;
}
default:
// 疑似错误的月份
return -1; // 或者抛出异常
}
}
// 计算特定日期是一年中的第几天
int day_of_year(int day, int month, int year) {
int total_days = 0;
for (int i = 1; i < month; ++i) {
total_days += days_in_month(i, year);
}
total_days += day;
return total_days;
}
int main() {
int year, month, day;
printf("请输入年月日(例如: 2023 2 1): ");
scanf("%d %d %d", &year, &month, &day);
if (day <= 0 || month <= 0 || month > 12 || day > days_in_month(month, year)) {
printf("无效的日期!\n");
} else {
int result = day_of_year(day, month, year);
printf("这一天是一年的第 %d 天。\n", result);
}
return 0;
}
```
当你运行此程序并提供有效的日期,它会计算并打印出该日期是一年中的第几天。注意,这个程序假设用户输入的月份和日期是合法的,并没有做额外的错误检查。
阅读全文
相关推荐
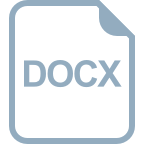
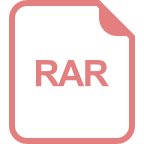
















