cpp 定义一个描述学生基本情况的类Student,数据成员包括姓名、学号、C++成绩、英语和数学成绩,成员函数包括输出所有信息函数(姓名、学号、各科成绩),求总成绩函数和求平均成绩。要求写出构造函数、拷贝构造函数,所有成员函数实现不要写在class大括号内。编写主函数测试该类。
时间: 2023-09-16 15:05:14 浏览: 85
### 回答1:
以下是一个基本的 C++ 类 `Student`,包括构造函数、析构函数、成员变量和成员函数,可以存储学生的基本信息(姓名、学号、各科成绩等),并计算总成绩和平均成绩。
```cpp
#include <iostream>
#include <string>
using namespace std;
class Student {
public:
Student(string name, int id, double cScore, double cppScore, double mathScore, double englishScore) {
this->name = name;
this->id = id;
this->cScore = cScore;
this->cppScore = cppScore;
this->mathScore = mathScore;
this->englishScore = englishScore;
}
~Student() {
cout << "Deleting student " << this->name << "..." << endl;
}
string getName() {
return this->name;
}
int getId() {
return this->id;
}
double getTotalScore() {
return this->cScore + this->cppScore + this->mathScore + this->englishScore;
}
double getAverageScore() {
return this->getTotalScore() / 4.0;
}
private:
string name;
int id;
double cScore;
double cppScore;
double mathScore;
double englishScore;
};
int main() {
Student s("张三", 1001, 90, 85, 92, 88);
cout << "姓名:" << s.getName() << endl;
cout << "学号:" << s.getId() << endl;
cout << "C++成绩:" << s.getTotalScore() << endl;
cout << "平均成绩:" << s.getAverageScore() << endl;
return 0;
}
```
为了实现成员函数的功能,我们需要将成员变量定义在 `private` 访问权限下,以保证数据的安全性。同时,我们需要定义公有的成员函数来访问和修改成员变量的值,以实现类的封装性。在构造函数中初始化成员变量的值,在析构函数中清理资源。
主函数中创建一个 `Student` 对象,并输出该学生的姓名、学号、总成绩和平均成绩。
值得注意的是,所有成员函数都不需要写在类的声明中,而是在类的定义外部实现。
### 回答2:
以下是一个简单的CPP代码,实现了描述学生基本情况的类Student:
```cpp
#include<iostream>
#include<string>
using namespace std;
class Student {
private:
string name;
string number;
double cGrade;
double englishGrade;
double mathGrade;
public:
Student(string n, string num, double cg, double eg, double mg);
Student(const Student& other);
void printInfo(); // 输出所有信息函数
double totalGrade(); // 求总成绩函数
double averageGrade(); // 求平均成绩函数
};
Student::Student(string n, string num, double cg, double eg, double mg) {
name = n;
number = num;
cGrade = cg;
englishGrade = eg;
mathGrade = mg;
}
Student::Student(const Student& other) {
name = other.name;
number = other.number;
cGrade = other.cGrade;
englishGrade = other.englishGrade;
mathGrade = other.mathGrade;
}
void Student::printInfo() {
cout << "姓名: " << name << endl;
cout << "学号: " << number << endl;
cout << "C成绩: " << cGrade << endl;
cout << "英语成绩: " << englishGrade << endl;
cout << "数学成绩: " << mathGrade << endl;
}
double Student::totalGrade() {
return cGrade + englishGrade + mathGrade;
}
double Student::averageGrade() {
return totalGrade() / 3;
}
int main() {
Student student1("张三", "2021001", 90, 85, 95);
Student student2 = student1;
student1.printInfo();
cout << "总成绩: " << student1.totalGrade() << endl;
cout << "平均成绩: " << student1.averageGrade() << endl;
student2.printInfo();
cout << "总成绩: " << student2.totalGrade() << endl;
cout << "平均成绩: " << student2.averageGrade() << endl;
return 0;
}
```
这个程序定义了一个名为Student的类,包含了有关学生的基本信息,如姓名、学号和各科成绩。构造函数用于初始化对象的成员变量,拷贝构造函数用于创建一个新的对象,其中成员变量的值与原始对象的成员变量相同。
类中的成员函数包括输出所有信息函数printInfo(),该函数用于输出学生的姓名、学号和各科成绩;求总成绩函数totalGrade(),该函数将各科成绩相加得到总成绩;求平均成绩函数averageGrade(),该函数将总成绩除以3得到平均成绩。
在主函数中,我们创建了两个学生对象student1和student2,并分别调用了各个成员函数,以测试这些函数的功能。输出结果将显示学生的基本信息、总成绩和平均成绩。
### 回答3:
下面是一个简单的使用C++定义一个描述学生基本情况的类Student,包括构造函数、拷贝构造函数、成员函数以及主函数的代码示例:
```cpp
#include <iostream>
#include <string>
using namespace std;
class Student {
string name;
int id;
int cScore;
int englishScore;
int mathScore;
public:
Student(string _name, int _id, int _cScore, int _englishScore, int _mathScore) {
name = _name;
id = _id;
cScore = _cScore;
englishScore = _englishScore;
mathScore = _mathScore;
}
Student(const Student& other) {
name = other.name;
id = other.id;
cScore = other.cScore;
englishScore = other.englishScore;
mathScore = other.mathScore;
}
void printInfo() {
cout << "姓名:" << name << " 学号:" << id << " C成绩:" << cScore << " 英语成绩:" << englishScore << " 数学成绩:" << mathScore << endl;
}
int getTotalScore() {
return cScore + englishScore + mathScore;
}
float getAverageScore() {
return (cScore + englishScore + mathScore) / 3.0;
}
};
int main() {
Student s1("张三", 1001, 90, 80, 95);
Student s2 = s1; // 使用拷贝构造函数来创建s2
s1.printInfo();
cout << "s1的总成绩:" << s1.getTotalScore() << endl;
cout << "s1的平均成绩:" << s1.getAverageScore() << endl;
s2.printInfo();
cout << "s2的总成绩:" << s2.getTotalScore() << endl;
cout << "s2的平均成绩:" << s2.getAverageScore() << endl;
return 0;
}
```
以上代码中,首先定义了一个`Student`类,包含了姓名、学号、C成绩、英语成绩和数学成绩这几个数据成员。在构造函数和拷贝构造函数中,通过传入的参数对成员变量进行初始化。成员函数`printInfo()`用于输出所有信息,`getTotalScore()`用于求总成绩,`getAverageScore()`用于求平均成绩。
在主函数中,创建了两个`Student`对象`s1`和`s2`,并分别调用成员函数来打印信息、求总成绩和平均成绩,以及使用拷贝构造函数将`s1`对象拷贝给`s2`对象。
相关推荐
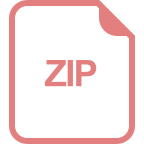
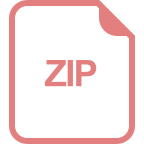
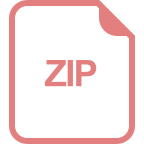
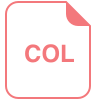
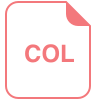
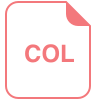
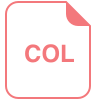
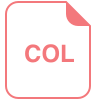









