定义一个Student类,其中包含学号,姓名,成绩等数据成员。建立若干个Student类对象,将他们保存到文件Record.dat中,然后显示文件中的内容
时间: 2023-12-02 15:02:50 浏览: 198
好的,这是一个典型的面向对象编程的问题,我可以为你提供一个C++的解决方案。
首先,我们需要定义一个Student类,其中包含学号,姓名,成绩等数据成员:
```cpp
class Student {
public:
int id; // 学号
string name; // 姓名
double score; // 成绩
};
```
然后,我们可以定义一个函数来读取多个Student对象,将它们保存到文件Record.dat中:
```cpp
void saveToFile(vector<Student>& students) {
ofstream outfile("Record.dat", ios::binary);
for (auto& s : students) {
outfile.write(reinterpret_cast<char*>(&s), sizeof(Student));
}
outfile.close();
}
```
其中,`vector<Student>& students`是一个保存了多个Student对象的向量。`ofstream`是一个用于写入文件的输出流,我们可以使用`write`函数将Student对象的二进制表示写入文件中。
最后,我们可以编写一个函数来读取Record.dat文件,并显示其中的内容:
```cpp
void displayFile() {
ifstream infile("Record.dat", ios::binary);
Student s;
while (infile.read(reinterpret_cast<char*>(&s), sizeof(Student))) {
cout << "ID: " << s.id << endl;
cout << "Name: " << s.name << endl;
cout << "Score: " << s.score << endl;
cout << endl;
}
infile.close();
}
```
在这个函数中,我们使用`ifstream`来读取Record.dat文件,并使用`read`函数将每个Student对象的二进制表示读入到`s`变量中。然后,我们可以输出每个Student对象的内容。
现在,我们可以编写一个简单的main函数来测试我们的代码:
```cpp
int main() {
vector<Student> students = {
{1, "Tom", 90.5},
{2, "Jerry", 85.0},
{3, "Alice", 92.5},
{4, "Bob", 88.0}
};
saveToFile(students);
displayFile();
return 0;
}
```
在这个main函数中,我们创建了四个Student对象,并将它们保存到Record.dat文件中。然后,我们调用displayFile函数,读取并显示Record.dat文件中的内容。
希望这个解决方案可以帮到你!
阅读全文
相关推荐
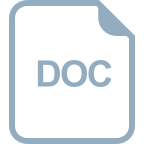
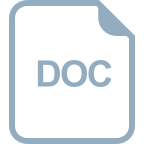
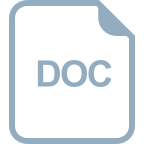








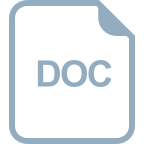
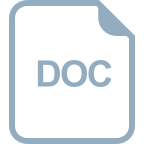
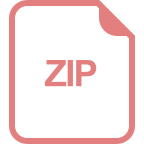
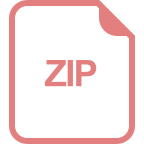
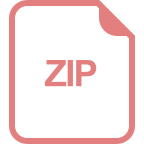