GO语言操作redis对geo类型进行curd
时间: 2023-08-20 09:05:18 浏览: 41
操作 Redis 的 GO 语言客户端有很多,比如 go-redis、redigo 等。下面是使用 go-redis 客户端对 Redis 中的 Geo 类型进行 CURD 操作的示例代码。
首先,需要先安装 go-redis 包:
```
go get github.com/go-redis/redis/v8
```
然后,在代码中导入 go-redis 包:
```go
import "github.com/go-redis/redis/v8"
```
接下来,就可以使用 go-redis 包提供的方法对 Redis 中的 Geo 类型进行 CURD 操作了。
**1. 连接 Redis**
```go
func connectRedis() *redis.Client {
rdb := redis.NewClient(&redis.Options{
Addr: "localhost:6379",
Password: "", // Redis 密码
DB: 0, // Redis 数据库
})
err := rdb.Ping(ctx).Err()
if err != nil {
panic(err)
}
return rdb
}
```
**2. 添加地理位置数据**
```go
func addGeoData(rdb *redis.Client) {
// 添加地理位置数据
err := rdb.GeoAdd(ctx, "cities", &redis.GeoLocation{
Name: "Beijing",
Longitude: 116.397128,
Latitude: 39.916527,
}).Err()
if err != nil {
panic(err)
}
}
```
**3. 获取地理位置数据**
```go
func getGeoData(rdb *redis.Client) {
// 获取地理位置数据
locations, err := rdb.GeoRadius(ctx, "cities", 116.397128, 39.916527, &redis.GeoRadiusQuery{
Radius: 100000, // 距离范围
Unit: "m", // 距离单位
WithGeoHash: true, // 是否返回 GeoHash 值
WithCoord: true, // 是否返回经纬度坐标
WithDist: true, // 是否返回距离
Count: 10, // 返回结果数量
}).Result()
if err != nil {
panic(err)
}
for _, location := range locations {
fmt.Printf("name: %s, longitude: %f, latitude: %f, distance: %f, geohash: %s\n",
location.Name, location.Longitude, location.Latitude, location.Distance, location.GeoHash)
}
}
```
**4. 删除地理位置数据**
```go
func deleteGeoData(rdb *redis.Client) {
// 删除地理位置数据
err := rdb.ZRem(ctx, "cities", "Beijing").Err()
if err != nil {
panic(err)
}
}
```
完整示例代码:
```go
package main
import (
"context"
"fmt"
"github.com/go-redis/redis/v8"
)
var ctx = context.Background()
func main() {
// 连接 Redis
rdb := connectRedis()
defer rdb.Close()
// 添加地理位置数据
addGeoData(rdb)
// 获取地理位置数据
getGeoData(rdb)
// 删除地理位置数据
deleteGeoData(rdb)
}
func connectRedis() *redis.Client {
rdb := redis.NewClient(&redis.Options{
Addr: "localhost:6379",
Password: "", // Redis 密码
DB: 0, // Redis 数据库
})
err := rdb.Ping(ctx).Err()
if err != nil {
panic(err)
}
return rdb
}
func addGeoData(rdb *redis.Client) {
// 添加地理位置数据
err := rdb.GeoAdd(ctx, "cities", &redis.GeoLocation{
Name: "Beijing",
Longitude: 116.397128,
Latitude: 39.916527,
}).Err()
if err != nil {
panic(err)
}
}
func getGeoData(rdb *redis.Client) {
// 获取地理位置数据
locations, err := rdb.GeoRadius(ctx, "cities", 116.397128, 39.916527, &redis.GeoRadiusQuery{
Radius: 100000, // 距离范围
Unit: "m", // 距离单位
WithGeoHash: true, // 是否返回 GeoHash 值
WithCoord: true, // 是否返回经纬度坐标
WithDist: true, // 是否返回距离
Count: 10, // 返回结果数量
}).Result()
if err != nil {
panic(err)
}
for _, location := range locations {
fmt.Printf("name: %s, longitude: %f, latitude: %f, distance: %f, geohash: %s\n",
location.Name, location.Longitude, location.Latitude, location.Distance, location.GeoHash)
}
}
func deleteGeoData(rdb *redis.Client) {
// 删除地理位置数据
err := rdb.ZRem(ctx, "cities", "Beijing").Err()
if err != nil {
panic(err)
}
}
```
相关推荐
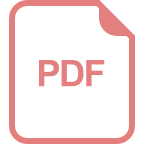














