飞机订票系统 c语言代码
时间: 2023-07-31 10:11:43 浏览: 113
以下是一个简单的飞机订票系统的C语言代码示例:
```c
#include <stdio.h>
#include <stdlib.h>
#include <string.h>
#define MAX_FLIGHTS 10
#define MAX_SEATS 50
struct flight {
int id;
char destination[50];
int seats[MAX_SEATS];
int num_seats;
int price;
};
struct flight flights[MAX_FLIGHTS];
int num_flights = 0;
void add_flight() {
if (num_flights >= MAX_FLIGHTS) {
printf("Maximum number of flights reached.\n");
return;
}
struct flight f;
f.id = num_flights + 1;
printf("Enter destination: ");
fgets(f.destination, 50, stdin);
f.destination[strcspn(f.destination, "\n")] = 0;
printf("Enter price: ");
scanf("%d", &f.price);
f.num_seats = MAX_SEATS;
for (int i = 0; i < MAX_SEATS; i++) {
f.seats[i] = 0;
}
flights[num_flights] = f;
num_flights++;
}
void show_flights() {
printf("Flights:\n");
for (int i = 0; i < num_flights; i++) {
printf("%d. %s, %d seats, $%d\n", flights[i].id, flights[i].destination, flights[i].num_seats, flights[i].price);
}
}
void book_seat() {
int flight_id, seat_num;
printf("Enter flight ID: ");
scanf("%d", &flight_id);
if (flight_id < 1 || flight_id > num_flights) {
printf("Invalid flight ID.\n");
return;
}
struct flight *f = &flights[flight_id - 1];
printf("Enter seat number: ");
scanf("%d", &seat_num);
if (seat_num < 1 || seat_num > MAX_SEATS) {
printf("Invalid seat number.\n");
return;
}
if (f->seats[seat_num - 1] == 1) {
printf("Seat already booked.\n");
return;
}
f->seats[seat_num - 1] = 1;
f->num_seats--;
printf("Seat booked successfully.\n");
}
int main() {
int choice;
do {
printf("\n1. Add flight\n2. Show flights\n3. Book seat\n4. Exit\n");
scanf("%d", &choice);
switch (choice) {
case 1:
add_flight();
break;
case 2:
show_flights();
break;
case 3:
book_seat();
break;
case 4:
printf("Goodbye!\n");
break;
default:
printf("Invalid choice.\n");
break;
}
} while (choice != 4);
return 0;
}
```
这个飞机订票系统可以添加航班、显示航班列表、预定座位等功能。每个航班可以有最多50个座位,每个座位可以被预定一次。在这个简单的示例中,航班信息存储在结构体数组中,每个航班有一个唯一的ID、目的地、价格和座位列表。
相关推荐
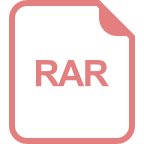








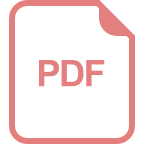
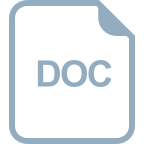
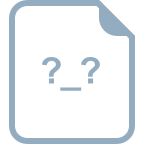