编程实现任意输入年月日计算出该天是当天的第几天
时间: 2024-05-07 09:23:48 浏览: 67
以下是 Python 代码实现:
```python
year = int(input("输入年份:"))
month = int(input("输入月份:"))
day = int(input("输入日期:"))
days_in_months = [31,28,31,30,31,30,31,31,30,31,30,31]
# 判断是否为闰年
if year % 4 == 0 and (year % 100 != 0 or year % 400 == 0):
days_in_months[1] = 29 # 闰年2月为29天
total_days = sum(days_in_months[:month-1]) + day
print("该天是当年的第{}天".format(total_days))
```
首先,用户输入年月日,然后定义一个列表 `days_in_months` 存储每个月的天数,其中默认2月为28天。接着,根据输入的年份判断是否为闰年,如果是,则将2月的天数修改为29天。最后,计算出当天是当年的第几天,并输出结果。
相关问题
编程实现任意输入年月日计算出该天是当年的第几天
以下是 Python 语言的实现代码:
```python
year = int(input("请输入年份:"))
month = int(input("请输入月份:"))
day = int(input("请输入日期:"))
# 计算该月之前的天数
days_in_previous_months = 0
for m in range(1, month):
if m in [1, 3, 5, 7, 8, 10, 12]:
days_in_previous_months += 31
elif m in [4, 6, 9, 11]:
days_in_previous_months += 30
else:
if (year % 4 == 0 and year % 100 != 0) or year % 400 == 0:
days_in_previous_months += 29
else:
days_in_previous_months += 28
# 加上当月的天数
days_in_current_month = day
# 加上该年之前的天数
if (year % 4 == 0 and year % 100 != 0) or year % 400 == 0:
days_in_year_before_current_month = 366
else:
days_in_year_before_current_month = 365
days_before_current_date = days_in_previous_months + days_in_current_month
# 输出结果
print("该天是当年的第{}天。".format(days_before_current_date))
```
你可以直接拷贝代码到 Python 环境中运行,也可以自己手动输入代码。其中,我们首先通过 `input` 函数获取用户输入的年、月、日信息,然后根据公历计算出该天是当年的第几天。
需要注意的是,该代码中的闰年判断采用的是公历闰年判断方式,即:若该年能被4整除但不能被100整除,或者能被400整除,则为闰年。
编程实现任意输入年月日,计算出该天是当年的第几天
好的,我可以编写一个简单的Python程序来实现这个功能。以下是完整的程序代码:
```python
year = int(input("请输入年份:"))
month = int(input("请输入月份:"))
day = int(input("请输入日期:"))
# 计算每个月份的天数
days_in_month = [31, 28, 31, 30, 31, 30, 31, 31, 30, 31, 30, 31]
# 判断是否为闰年
if (year % 4 == 0 and year % 100 != 0) or (year % 400 == 0):
days_in_month[1] = 29
# 计算当天是该年的第几天
day_count = sum(days_in_month[:month-1]) + day
print("该天是当年的第 %d 天。" % day_count)
```
程序首先要求用户输入年份、月份和日期。然后,程序计算每个月份的天数,并根据输入的年份判断是否为闰年。最后,程序使用列表切片和sum函数计算出当天是该年的第几天,并输出结果。
希望这个程序能够帮助到你!
阅读全文
相关推荐
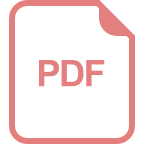
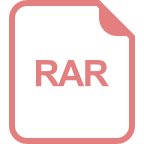

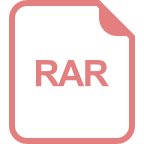
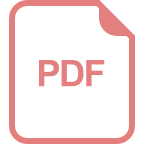
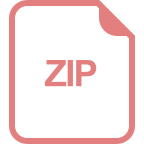
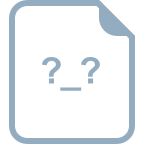
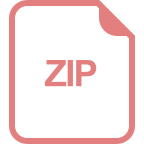
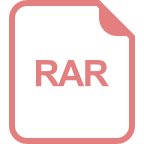
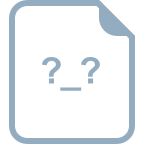
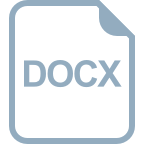
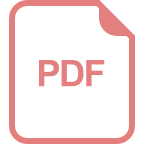
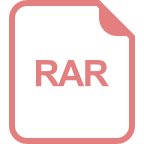
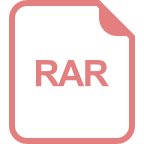
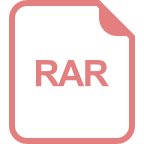
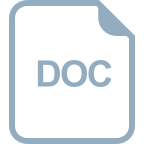