java如何使乱序的字符变成常序
时间: 2024-09-09 21:04:39 浏览: 39
在Java中,如果你想将乱序的字符数组变为有序,可以使用各种排序算法,例如冒泡排序、插入排序、选择排序、快速排序、归并排序等。这里以快速排序为例,它是一种常用的高效排序算法:
```java
public class SortChars {
public static void quickSort(char[] chars, int low, int high) {
if (low < high) {
// 分治策略
int pivotIndex = partition(chars, low, high);
quickSort(chars, low, pivotIndex - 1); // 对左半部分递归排序
quickSort(chars, pivotIndex + 1, high); // 对右半部分递归排序
}
}
private static int partition(char[] chars, int low, int high) {
char pivot = chars[high];
int i = low - 1;
for (int j = low; j < high; j++) {
if (chars[j] <= pivot) {
i++;
swap(chars, i, j);
}
}
swap(chars, i + 1, high); // 将pivot放到正确的位置
return i + 1;
}
private static void swap(char[] chars, int i, int j) {
char temp = chars[i];
chars[i] = chars[j];
chars[j] = temp;
}
public static void main(String[] args) {
char[] unsortedChars = {'z', 'a', 'c', 'b'};
quickSort(unsortedChars, 0, unsortedChars.length - 1);
System.out.println("Sorted array: " + Arrays.toString(unsortedChars));
}
}
```
运行这个程序后,输入的乱序字符数组会变成升序排列。
阅读全文
相关推荐
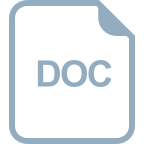
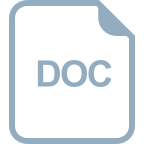
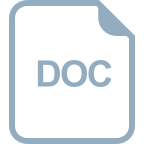

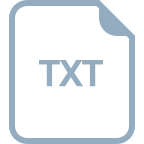
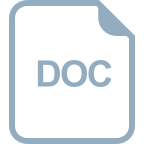
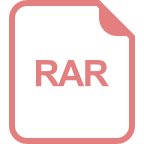



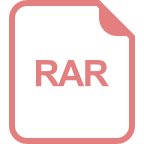
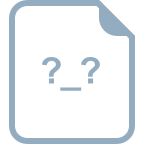
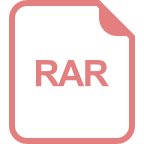
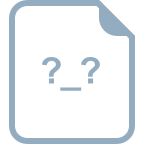
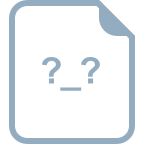