vue3.2父组件调用子组件的方法
时间: 2023-09-21 09:07:07 浏览: 134
在Vue 3.2中,父组件可以通过使用`ref`或`$refs`来获取子组件的实例,进而调用子组件的方法。
首先,在子组件中,需要使用`ref`属性给子组件的实例命名。例如,在子组件的模板上,可以添加`ref="childComponent"`来给子组件的实例命名为`childComponent`。
然后,在父组件中,可以使用`ref`或`$refs`来获取子组件的实例。如果父组件使用了`ref`来命名子组件的实例,可以直接使用`this.$refs.childComponent`来获取子组件的实例。如果父组件没有使用`ref`来命名子组件的实例,可以使用`this.$refs`来获取一个对象,其中包含了所有被命名的子组件实例。然后,可以通过`this.$refs.childComponent`来访问子组件的实例。
一旦获取了子组件的实例,就可以调用子组件的方法了。例如,如果子组件有一个名为`doSomething`的方法,可以使用`this.$refs.childComponent.doSomething()`来调用子组件的方法。
请注意,使用`ref`或`$refs`来获取子组件的实例需要确保子组件已经被渲染到DOM中。如果尝试在子组件渲染之前获取子组件的实例,将会得到`undefined`。
综上所述,在Vue 3.2中,父组件可以通过使用`ref`或`$refs`来获取子组件的实例,并调用子组件的方法。
相关问题
vue3.2 父组件调用子组件方法
在 Vue 3.2 中,你可以通过使用 `ref` 和 `teleport` 来实现父组件调用子组件的方法。下面是一个示例:
在子组件中,你需要使用 `ref` 来创建一个引用,并将它暴露给父组件。然后,在子组件中,你可以通过 `teleport` 将子组件的方法绑定到引用上。这样,父组件就可以通过引用来调用子组件的方法了。
下面是一个示例代码:
// 子组件 Child.vue
<template>
<div>
<button @click="childMethod">子组件方法</button>
</div>
</template>
<script>
import { ref, teleport } from 'vue';
export default {
setup() {
const childMethod = () => {
console.log('子组件方法被调用了');
};
const childMethodRef = ref(childMethod);
teleport(() => {
return { childMethodRef };
});
return {
childMethod
};
}
};
</script>
在父组件中,你可以通过 `$refs` 来访问子组件的引用,并调用子组件的方法。
// 父组件 Parent.vue
<template>
<div>
<button @click="callChildMethod">调用子组件方法</button>
<child ref="childRef"></child>
</div>
</template>
<script>
import { ref } from 'vue';
export default {
setup() {
const childRef = ref(null);
const callChildMethod = () => {
if (childRef.value) {
childRef.value.childMethodRef();
}
};
return {
callChildMethod
};
}
};
</script>
在上面的示例中,我们在父组件中创建了一个子组件的引用 `childRef`,然后通过 `this.$refs.childRef.childMethodRef()` 调用子组件的方法。
注意:在 Vue 3 中,需要使用 `ref` 来创建引用,而不是像 Vue 2 中那样使用 `$refs`。
VUE3.2 父组件怎么调用子组件的方法
在 Vue 3.2 中,您可以通过 `ref` 和 `defineExpose` 来访问子组件的方法。
首先,在子组件中,您需要使用 `defineExpose` 函数来暴露需要向父组件公开的方法。例如:
```vue
<template>
<div>{{ count }}</div>
</template>
<script>
import { defineComponent } from 'vue'
export default defineComponent({
data() {
return {
count: 0
}
},
methods: {
increment() {
this.count++
},
decrement() {
this.count--
},
reset() {
this.count = 0
},
// 使用 defineExpose 函数暴露 increment 方法
// 注意,只有被暴露的属性和方法才能被父组件访问
defineExpose() {
return {
increment: this.increment
}
}
}
})
</script>
```
在上面的示例中,我们使用 `defineExpose` 函数暴露了 `increment` 方法,该方法会增加 `count` 属性的值。
然后,在父组件中,您可以使用 `ref` 来获取子组件实例,并通过该实例来调用子组件的方法。例如:
```vue
<template>
<div>
<button @click="handleClick">调用子组件的 increment 方法</button>
</div>
<child-component ref="child"></child-component>
</template>
<script>
import { ref } from 'vue'
import ChildComponent from './ChildComponent.vue'
export default {
components: {
ChildComponent
},
setup() {
const childRef = ref(null)
const handleClick = () => {
childRef.value.increment()
}
return {
childRef,
handleClick
}
}
}
</script>
```
在上面的示例中,我们首先使用 `ref` 函数创建了一个子组件的引用 `childRef`,并在父组件的 `setup` 函数中定义了一个 `handleClick` 函数,该函数通过 `childRef.value` 获取子组件实例,并调用子组件暴露的 `increment` 方法来增加 `count` 属性的值。在模板中,我们使用 `handleClick` 来调用子组件的 `increment` 方法。
注意,只有在子组件中使用 `defineExpose` 函数暴露的属性和方法才能被父组件访问。如果子组件中没有使用 `defineExpose` 函数来暴露方法,父组件将无法访问子组件的方法。同时,`defineExpose` 函数返回的对象中,只有被暴露的属性和方法才能被父组件访问,未被暴露的属性和方法将不会被父组件访问到。
阅读全文
相关推荐
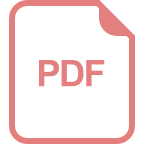
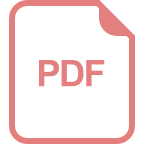
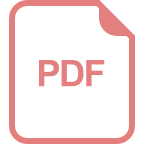













