如何在C++中实现从文本文件读取单词并统计频率?请提供一个详细的程序设计和代码实现。
时间: 2024-11-01 21:24:25 浏览: 46
要实现从文本文件中读取单词并统计频率的功能,首先需要熟悉C++的文件I/O操作,链表数据结构,以及字符串的处理方法。《C++程序实现单词频率统计》为你提供了一个综合性的项目实战案例,涵盖了从文件读取到单词频率统计的完整流程。
参考资源链接:[C++程序实现单词频率统计](https://wenku.csdn.net/doc/30nigwnpgb?spm=1055.2569.3001.10343)
具体实现步骤如下:
1. 定义一个`word`类,包含单词和频率的存储,以及相关的函数成员,如添加单词到链表、查找单词、显示频率等。
2. 使用`fstream`库中的`ifstream`对象打开并读取指定的文本文件,逐行读取并去除标点符号,然后将清理后的单词添加到链表中。
3. 设计一个链表结构,用于存储文件中读取的单词和对应频率。链表的每个节点是一个`word`对象。
4. 实现`searchword`函数,遍历链表以查找特定单词,并在找到时返回其频率。
5. 实现`frequencydisplay`函数,遍历链表中所有不重复的单词,使用`searchword`函数计算每个单词的频率,并在控制台输出。
以下是一个简化版的代码示例,展示了如何实现上述功能:
```cpp
#include <iostream>
#include <fstream>
#include <string>
#include <cctype>
#include <list>
#include <algorithm>
class Word {
friend void frequencyDisplay(std::list<Word>& words);
friend void searchWord(std::list<Word>& words, const std::string& targetWord);
private:
std::string word;
int frequency;
Word* next;
public:
Word(const std::string& w) : word(w), frequency(1), next(nullptr) {}
~Word() {}
};
void removePunctuation(std::string& word) {
std::replace_if(word.begin(), word.end(),ispunct, ' ');
}
void openFile(std::list<Word>& words, const std::string& filename) {
std::ifstream file(filename);
std::string line, word;
while(getline(file, line)) {
removePunctuation(line);
std::istringstream iss(line);
while(iss >> word) {
words.push_back(word);
}
}
}
void frequencyDisplay(std::list<Word>& words) {
for (const auto& w : words) {
std::cout << w.word <<
参考资源链接:[C++程序实现单词频率统计](https://wenku.csdn.net/doc/30nigwnpgb?spm=1055.2569.3001.10343)
阅读全文
相关推荐
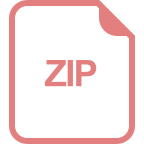
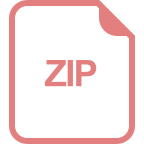
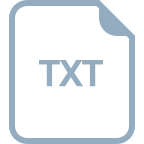

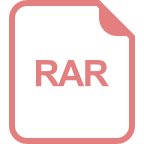
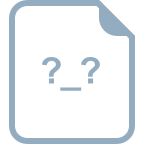
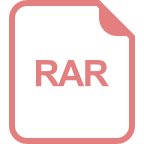
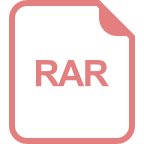
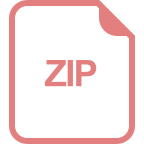
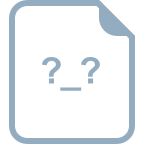
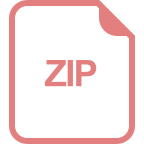
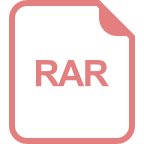
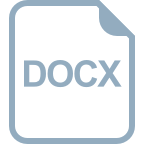
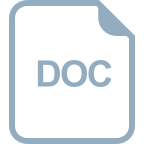
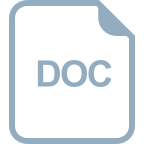
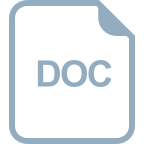
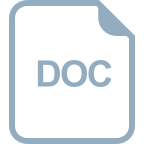
