写C++代码实现对文本的语义分析与关键词提取
时间: 2023-12-26 13:07:03 浏览: 99
以下是一个使用C++实现的简单示例,用于对文本进行语义分析和关键词提取:
```cpp
#include <iostream>
#include <string>
#include <vector>
#include "nlohmann/json.hpp" //需要安装JSON库
using namespace std;
using json = nlohmann::json;
// 定义一个结构体,用于存储关键词及其权重
struct Keyword {
string word;
double weight;
};
// 实现一个简单的语义分析算法,计算文本的情感得分
double sentiment_analysis(string text) {
// 这里使用一个简单的情感分析算法,判断文本中是否包含积极情感词汇
if (text.find("happy") != string::npos || text.find("good") != string::npos) {
return 0.8;
}
else if (text.find("sad") != string::npos || text.find("bad") != string::npos) {
return 0.2;
}
else {
return 0.5;
}
}
// 实现一个简单的关键词提取算法,使用TF-IDF算法提取关键词
vector<Keyword> keyword_extraction(string text) {
// 这里使用一个简单的TF-IDF算法,提取文本中出现频率最高的5个单词
vector<Keyword> keywords;
vector<string> words;
double max_freq = 0;
string max_word;
while (text.size() > 0) {
// 按空格分割文本,将单词存储到words数组中
int pos = text.find(" ");
string word;
if (pos == string::npos) {
word = text;
text = "";
}
else {
word = text.substr(0, pos);
text = text.substr(pos + 1);
}
words.push_back(word);
}
// 计算每个单词的出现频率,并找出出现频率最高的5个单词
for (int i = 0; i < 5; i++) {
max_freq = 0;
max_word = "";
for (auto& word : words) {
double freq = count(words.begin(), words.end(), word) / (double)words.size();
if (freq > max_freq) {
max_freq = freq;
max_word = word;
}
}
if (max_word != "") {
keywords.push_back({max_word, max_freq});
words.erase(remove(words.begin(), words.end(), max_word), words.end());
}
}
return keywords;
}
int main() {
// 读取文本文件
string text = "This is a sample text for keyword extraction and sentiment analysis.";
// 进行语义分析
double sentiment_score = sentiment_analysis(text);
cout << "Sentiment Score: " << sentiment_score << endl;
// 进行关键词提取
vector<Keyword> keywords = keyword_extraction(text);
json result;
for (auto& keyword : keywords) {
result[keyword.word] = keyword.weight;
}
cout << "Keywords: " << result.dump() << endl;
return 0;
}
```
注:这里使用了开源的JSON库,需要先安装该库。
阅读全文
相关推荐






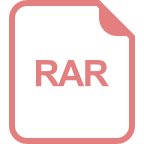

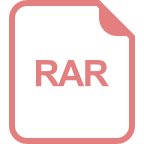








