使用python实现文本清洗、关键词提取、可视化
时间: 2023-10-20 11:18:12 浏览: 197
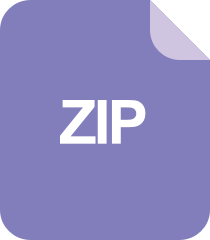
基于Python实现中文文本关键词抽取【100011007】
好的,下面是一个简单的示例:
## 文本清洗
我们先定义一个函数,用于对文本进行清洗。这里我们使用正则表达式来去除一些特殊字符和标点符号。
```python
import re
def clean_text(text):
# 去除特殊字符
text = re.sub(r'\W', ' ', text)
# 去除标点符号
text = re.sub(r'\s+', ' ', text)
# 去除多余空格
text = re.sub(r'\s+', ' ', text).strip()
return text
```
## 关键词提取
接下来,我们使用`gensim`库来提取文本的关键词。
```python
from gensim.summarization import keywords
def extract_keywords(text):
return keywords(text).split('\n')
```
## 可视化
最后,我们使用`matplotlib`库来可视化关键词的出现频率。
```python
import matplotlib.pyplot as plt
def plot_keywords(keywords):
freq = {}
for word in keywords:
if word in freq:
freq[word] += 1
else:
freq[word] = 1
sorted_freq = sorted(freq.items(), key=lambda x: x[1], reverse=True)[:10]
plt.bar(range(len(sorted_freq)), [x[1] for x in sorted_freq], tick_label=[x[0] for x in sorted_freq])
plt.show()
```
完整的示例代码如下:
```python
import re
from gensim.summarization import keywords
import matplotlib.pyplot as plt
def clean_text(text):
# 去除特殊字符
text = re.sub(r'\W', ' ', text)
# 去除标点符号
text = re.sub(r'\s+', ' ', text)
# 去除多余空格
text = re.sub(r'\s+', ' ', text).strip()
return text
def extract_keywords(text):
return keywords(text).split('\n')
def plot_keywords(keywords):
freq = {}
for word in keywords:
if word in freq:
freq[word] += 1
else:
freq[word] = 1
sorted_freq = sorted(freq.items(), key=lambda x: x[1], reverse=True)[:10]
plt.bar(range(len(sorted_freq)), [x[1] for x in sorted_freq], tick_label=[x[0] for x in sorted_freq])
plt.show()
text = """
Python 是一种面向对象、解释型计算机程序设计语言,由荷兰人 Guido van Rossum 于 1989 年发明,第一个公开发行版发行于 1991 年。Python 语法简洁清晰,具有丰富和强大的类库。它常被称为胶水语言,能够把用其他语言制作的各种模块(尤其是 C/C++)很轻松地联结在一起。Python 适用于许多领域,如网络编程、游戏编程、科学计算、人工智能等。
"""
cleaned_text = clean_text(text)
keywords = extract_keywords(cleaned_text)
plot_keywords(keywords)
```
运行后,会生成一个柱状图,显示文本中出现频率最高的关键词。
阅读全文
相关推荐
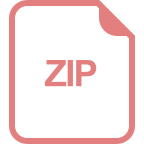
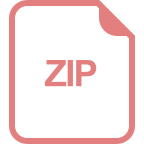
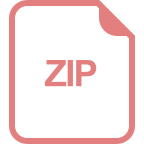
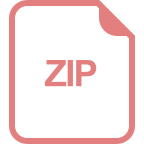
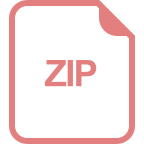
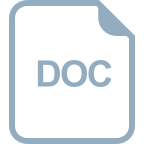
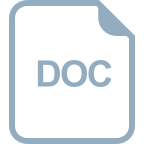
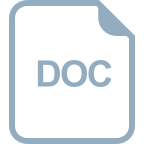
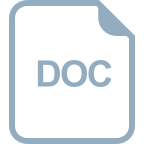
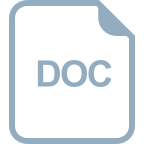
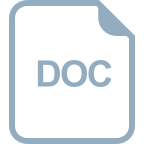
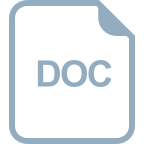
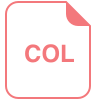
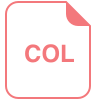
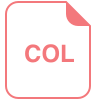

