C语言中使用后插法创建单链表的代码
时间: 2024-09-29 09:04:30 浏览: 40
在C语言中,后插法通常用于在已有的链表末尾添加新的节点。下面是一个简单的示例,展示如何通过指针操作实现这个过程:
```c
#include <stdio.h>
// 定义链表节点结构体
typedef struct Node {
int data;
struct Node* next; // 指向下一个节点的指针
} Node;
// 创建新节点并插入到链表末尾
void insertAtEnd(Node** head, int value) {
Node* newNode = (Node*)malloc(sizeof(Node)); // 分配内存
if (newNode == NULL) { // 如果分配失败,处理错误
printf("Memory allocation failed.\n");
return;
}
newNode->data = value;
newNode->next = NULL; // 新节点默认无前驱
// 如果链表为空或只有一个节点
if (*head == NULL || (*head)->next == NULL) {
*head = newNode; // 将新节点设置为首节点
} else {
Node* current = *head;
while (current->next != NULL) { // 遍历至链表末尾
current = current->next;
}
current->next = newNode; // 将新节点接到末尾
}
}
// 打印链表
void printList(Node* head) {
Node* temp = head;
while (temp != NULL) {
printf("%d -> ", temp->data);
temp = temp->next;
}
printf("NULL\n");
}
int main() {
Node* head = NULL;
insertAtEnd(&head, 1); // 插入第一个元素
insertAtEnd(&head, 2);
insertAtEnd(&head, 3);
printList(head); // 输出: 1 -> 2 -> 3 -> NULL
return 0;
}
```
在这个例子中,`insertAtEnd`函数接受一个指向头结点的指针,并在链表尾部追加新节点。如果链表为空,新节点就成为头结点。
阅读全文
相关推荐
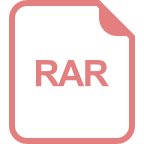
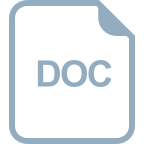




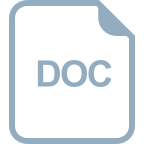
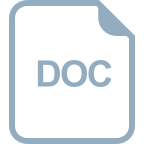
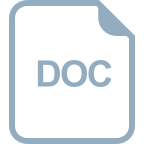
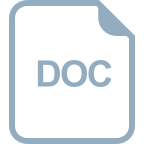



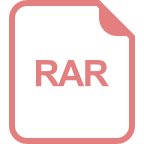