用C语言写一个单链表,要求用后插法
时间: 2023-05-19 12:05:41 浏览: 47
非常好的问题!以下是一个用C语言实现的单链表,使用后插法:
```c
#include <stdio.h>
#include <stdlib.h>
// 定义链表节点结构体
typedef struct node {
int data;
struct node *next;
} Node;
// 定义链表结构体
typedef struct list {
Node *head;
Node *tail;
} List;
// 初始化链表
void initList(List *list) {
list->head = NULL;
list->tail = NULL;
}
// 后插法插入节点
void insertNode(List *list, int data) {
Node *newNode = (Node *)malloc(sizeof(Node));
newNode->data = data;
newNode->next = NULL;
if (list->head == NULL) {
list->head = newNode;
list->tail = newNode;
} else {
list->tail->next = newNode;
list->tail = newNode;
}
}
// 打印链表
void printList(List *list) {
Node *current = list->head;
while (current != NULL) {
printf("%d ", current->data);
current = current->next;
}
printf("\n");
}
int main() {
List list;
initList(&list);
insertNode(&list, 1);
insertNode(&list, 2);
insertNode(&list, 3);
printList(&list);
return 0;
}
```
希望这个代码能够帮助你!
相关推荐
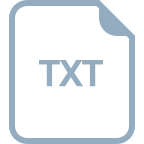














