用c语言按一下要求写一篇代码1. 请以“后插法”创建一个数据元素为整数的单链表。 2. 请编写一个函数可以让我自己插入单链表中的正数并打印。 3. 请编写一个Max函数获取单链表中包含的最大整数值。
时间: 2024-10-16 21:06:34 浏览: 7
好的,下面是使用C语言按照要求实现的代码:
```c
#include <stdio.h>
#include <stdlib.h>
// 定义链表节点结构体
typedef struct Node {
int data;
struct Node* next;
} Node;
// 创建链表头节点
Node* createList() {
return (Node*)malloc(sizeof(Node));
}
// 插入函数,使用后插法在链表尾部插入整数
void insert(int value) {
Node* newNode = (Node*)malloc(sizeof(Node)); // 创建新节点
newNode->data = value; // 设置数据
newNode->next = NULL; // 初始化下一个指针
if (head == NULL) { // 如果链表为空
head = newNode; // 新节点成为头节点
} else { // 链表非空
Node* temp = head;
while (temp->next != NULL) { // 找到最后一个节点
temp = temp->next;
}
temp->next = newNode; // 将新节点添加到末尾
}
}
// 打印链表中的所有数字
void printList(Node* node) {
while (node != NULL) {
printf("%d ", node->data);
node = node->next;
}
printf("\n");
}
// 获取最大值函数
int maxInList(Node* node) {
int max = node->data;
Node* temp = node->next;
while (temp != NULL) {
if (temp->data > max) {
max = temp->data;
}
temp = temp->next;
}
return max;
}
int main() {
Node* head = createList(); // 初始化空链表
insert(5); // 插入第一个元素
insert(10);
insert(3);
printf("Original list: ");
printList(head);
int userInput;
printf("Enter a positive number to insert: ");
scanf("%d", &userInput);
insert(userInput);
printf("After insertion: ");
printList(head);
int maxVal = maxInList(head);
printf("The maximum value in the list is: %d\n", maxVal);
return 0;
}
```
这个程序首先创建了一个空的单链表,然后用户可以输入正数插入链表,并打印出链表内容以及其中的最大值。
阅读全文
相关推荐
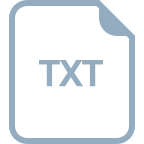
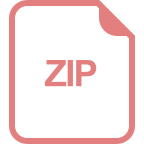















